Top Laravel Mistakes to Avoid for Smoother Development
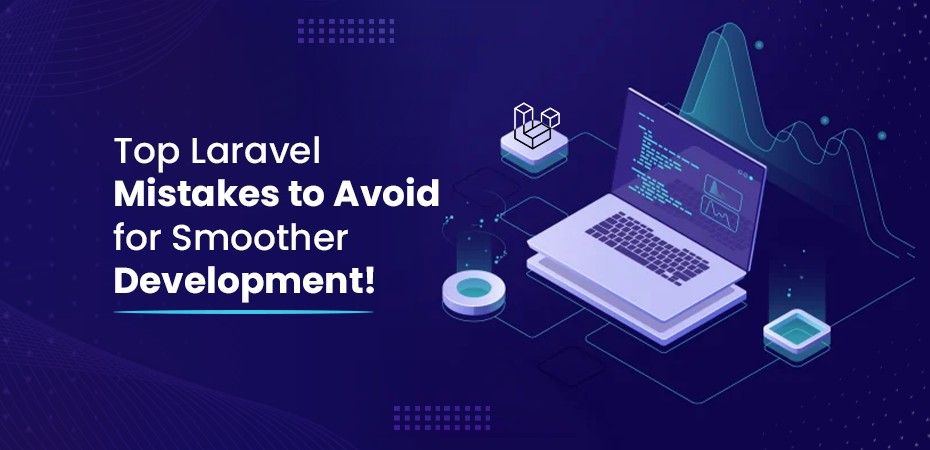
Laravel stands out as one of the most popular PHP frameworks, known for its elegant syntax and robust features. It simplifies many common tasks in web development, making it a favorite among developers. However, to harness the full potential of Laravel, it’s crucial to adhere to best practices throughout the development process.
Following these Laravel best practices in Laravel development ensures:
- Enhanced performance: Optimized code and efficient database queries.
- Improved security: Protection against common vulnerabilities.
- Better maintainability: Clean and organized codebase.
- Seamless collaboration: Consistent coding standards across teams.
This article dives into key bad practices that can derail your Laravel projects and offers insights on how to avoid them. From addressing the N+1 query problem to securing your Laravel applications, we cover essential tips for a seamless development experience.
Key Takeaways:
- Understand how to optimize database interactions.
- Learn strategies for securing your Laravel applications.
- Discover best practices for API development.
- Gain insights into maintaining a clean and organized codebase.
- Explore caching strategies to enhance performance.
By recognizing and addressing these pitfalls, you can significantly improve both the performance and maintainability of your Laravel applications.
Avoiding Common Laravel Bad Practices
N+1 Query Problem
The N+1 query problem is a common pitfall in Laravel development that can significantly degrade application performance. This issue occurs when your code executes multiple database queries because of improper handling of Eloquent relationships. For instance, imagine you have a Post model with a Comment relationship:
php $posts = Post::all(); foreach ($posts as $post) { echo $post->comments; }
In this scenario, if you have 100 posts, the above code will execute 1 query to fetch all posts and an additional 100 queries to fetch comments for each post, leading to a total of 101 queries.
Importance of Eager Loading
To mitigate the N+1 query problem, it is crucial to use eager loading. By utilizing the with() method, you can load all related data in a single query:
php $posts = Post::with(‘comments’)->get(); foreach ($posts as $post) { echo $post->comments; }
This approach drastically reduces the number of queries, enhancing performance and efficiency.
Excessive Database Loading
Loading more data than necessary can slow down your application. Developers often fall into the trap of fetching entire records when only a subset of data is required. Using methods like withCount() helps in retrieving only the essential data:
php $posts = Post::withCount(‘comments’)->get(); foreach ($posts as $post) { echo $post->comments_count; }
This method avoids loading full comment objects, thereby conserving resources.
Chaining Eloquent Methods Without Checks
Chaining Eloquent methods without proper checks poses significant risks. Consider the following example:
php $user = User::find($id)->posts()->first()->comments()->get();
If any intermediate object (User, Post) does not exist, this chain will throw an error. Incorporating checks prevents such issues:
php $user = User::find($id); if ($user) { $firstPost = $user->posts()->first(); if ($firstPost) { $comments = $firstPost->comments()->get(); } }
This ensures your code gracefully handles null values and avoids unexpected errors.
By understanding and addressing these bad practices, you can significantly improve the performance and reliability of your Laravel applications. Each practice highlighted here addresses specific pitfalls that developers encounter, providing actionable strategies for improvement.
Securing Your Laravel Applications
Common Security Issues in Laravel
Laravel, while robust and secure, is not immune to common security vulnerabilities. Recognizing and addressing these issues is crucial for maintaining the integrity of your application.
Some of the prevalent security threats include:
- SQL Injection: Unsanitized user inputs can manipulate SQL queries, leading to data breaches.
- Cross-Site Scripting (XSS): Malicious scripts injected into web pages can compromise user data.
- Cross-Site Request Forgery (CSRF): Unauthorized commands transmitted from a user that the web application trusts.
Validating and Sanitizing User Inputs
One effective way to mitigate these threats is by validating and sanitizing user inputs. Laravel provides several tools to accomplish this:
- Validation: Use Laravel’s built-in validation rules within your controllers or request classes. For example: php $validatedData = $request->validate([ ’email’ => ‘required|email’, ‘age’ => ‘required|integer|min:18’ ]);
- Sanitization: Ensure inputs are sanitized before processing them. Laravel’s e() helper function can escape HTML entities to prevent XSS attacks. php {!! e($userInput) !!}
By combining validation and sanitization, you can significantly reduce the risk of SQL injection and XSS attacks.
Implementing CSRF Protection
Cross-Site Request Forgery protection is another critical aspect of securing Laravel applications. Laravel comes with CSRF protection enabled by default:
- CSRF Tokens: Every form submission includes a CSRF token that verifies the request’s legitimacy. The @csrf directive in Blade templates simplifies this process: html.
- Verifying Tokens: Laravel automatically checks for the CSRF token in incoming requests, ensuring they originate from your application.
Understanding and implementing these security measures can protect your Laravel applications from various vulnerabilities, enhancing their overall resilience against malicious activities.
Best Practices for API Development in Laravel
Some of the Laravel API best practices in 2024 include:
Proper Use of HTTP Status Codes
Accurate HTTP status codes are crucial in API development. They communicate clearly with the client about the outcome of their request. Here are some guidelines:
- 200 OK: The request was successful.
- 201 Created: A new resource has been created successfully.
- 204 No Content: The request was successful, but there is no content to send back.
- 400 Bad Request: The server cannot process the request due to client error.
- 401 Unauthorized: Authentication is required and has failed or has not yet been provided.
- 403 Forbidden: The server understands the request but refuses to authorize it.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: A generic error message, given when an unexpected condition was encountered.
Using these status codes appropriately ensures clients can handle responses correctly and improve the user experience.
Handling Errors Gracefully
Effective error handling is crucial for robust APIs. Here are some Laravel best practices 2024:
Validation Errors:
- Use Laravel’s built-in validation system to catch errors early.
- Return a 422 Unprocessable Entity status code along with detailed error messages.
php $validator = Validator::make($request->all(), [ ’email’ => ‘required|email’, ‘password’ => ‘required|min:6’, ]);
if ($validator->fails()) { return response()->json([ ‘errors’ => $validator->errors() ], 422); }
Exception Handling:
- Customize exception handling in the Handler class (app/Exceptions/Handler.php).
- Provide user-friendly error messages without exposing sensitive information.
Standardized Error Responses:
- Ensure consistency by returning structured error responses.
- php return response()->json([ ‘error’ => [ ‘message’ => ‘Resource not found’, ‘code’ => 404 ] ], 404);
Logging Errors:
- Log errors using Laravel’s logging facilities to keep track of issues and for debugging purposes.
By following these best practices, you can create APIs that are reliable, maintainable, and user-friendly, ensuring a positive developer experience and effective client interaction.
Ensuring Code Quality and Organization in Laravel Projects
Maintaining a clean and organized codebase is essential for the long-term success of any Laravel project. Adhering to Laravel coding standards ensures that your code is not only readable but also maintainable, making collaboration with other developers smoother and more efficient.
Importance of Clean Code
Clean code isn’t just about aesthetics; it’s about functionality and efficiency. A well-organized codebase:
- Reduces Bugs: Clear and concise code helps in identifying errors quickly.
- Enhances Performance: Optimized code can lead to faster execution times.
- Facilitates Maintenance: Easier to update or refactor without introducing new issues.
- Improves Collaboration: Developers can understand each other’s work without extensive explanations.
Best Practices for Code Organization
Following Laravel coding best practices for code organization in Laravel projects can significantly improve your development workflow:
- Follow PSR Standards: Use PSR-1, PSR-2, and PSR-12 standards to ensure consistency in coding style. Employ tools like PHP_CodeSniffer to automatically check adherence.
- Utilize MVC Structure Effectively: This is one of the best Laravel controller best practices. Separate concerns by keeping business logic within models, application logic in controllers, and presentation logic within views. Avoid placing database queries or complex logic inside Blade templates.
- Adopt Naming Conventions: Use clear and descriptive names for classes, methods, and variables. Follow Laravel’s naming conventions for files and directories.
- Leverage Service Classes: Move reusable business logic into service classes rather than bloating controllers with excessive methods. This aligns with the Single Responsibility Principle (SRP) of software design.
- Implement Dependency Injection: Utilize Laravel’s IoC container to manage dependencies, promoting loose coupling between components. This approach simplifies testing and improves the scalability of your application.
- Use Repositories: Encapsulate data access logic within repository classes. This abstraction layer makes your application more flexible and easier to maintain.
Key Tools
The Laravel security best practices aid in maintaining high code quality:
- Laravel Pint: A robust PHP linter tailored for Laravel projects.
- PHPStan: A static analysis tool to catch potential bugs before runtime.
- PHPMD (PHP Mess Detector): Analyzes your code for possible issues like unused parameters or overly complex functions.
By integrating these tools into your development workflow, you can ensure that your Laravel projects remain clean, organized, and efficient.
Optimizing Database Interactions in Laravel Applications
Optimizing database interactions is crucial for ensuring efficient performance in Laravel applications. One of the primary considerations is choosing between raw SQL queries and Eloquent ORM.
Raw SQL Queries vs Eloquent ORM
Raw SQL Queries:
- Performance: Generally faster than Eloquent ORM because they are executed directly by the database engine without any abstraction layer.
- Flexibility: Allows for complex queries that might be cumbersome to construct using an ORM.
- Risk: Higher susceptibility to SQL injection attacks if user inputs are not properly sanitized.
Eloquent ORM:
- Readability: Provides a more readable and expressive syntax, making it easier to understand and maintain.
- Security: Automatically handles SQL injection protection, reducing security risks.
- Convenience: Simplifies common tasks such as relationships, pagination, and eager loading.
Best Practices
The Laravel design patterns and best practices are as follows:
- Use Eloquent for Simpler Queries: Ideal for CRUD operations where readability and maintainability are priorities.
- Opt for Raw Queries When Necessary: For complex queries or performance-critical paths, raw SQL might be more efficient.
- Prevent N+1 Query Problem with Eager Loading: Use with() method to load relationships alongside the main query to avoid multiple unnecessary database hits. php $users = User::with(‘posts’)->get();
- Minimize Data Loading: Fetch only required columns using methods like select() or withCount(). php $users = User::select(‘id’, ‘name’)->withCount(‘posts’)->get();
Balancing these strategies can significantly improve the performance and maintainability of your Laravel applications. Efficient database interaction is a cornerstone of scalable web applications, making it essential to choose the right approach based on the specific needs of your project.
Managing Configuration Files Effectively in Laravel Projects
Managing configuration files efficiently is crucial for maintaining a clean and flexible Laravel project. Here are some Laravel testing best practices:
Avoid Hardcoding Values
Hardcoding values directly in your code can lead to maintenance headaches and inflexibility. Instead, use the configuration files located in the config directory. This approach ensures that changes can be made in one place and propagates throughout the application.
php // Instead of this: $apiKey = ‘your-api-key-here’;
// Do this: $apiKey = config(‘services.api.key’);
Utilize Environment Variables
Environment variables, defined in the .env file, provide another layer of flexibility and security by keeping sensitive information out of your source code.
env // .env file API_KEY=your-api-key-here
php // Accessing environment variable in config file ‘key’ => env(‘API_KEY’),
Categorize Configuration Settings
Organize configuration settings logically by creating custom configuration files if necessary. For example, you might have a separate config/payment.php for payment-related settings.
Leverage Laravel Enums Best Practices
For managing enumerations, consider using enums to ensure type safety and reduce errors. You can utilize packages like spatie/enum for better enum management.
Config Caching for Performance
Use Laravel’s config:cache command to cache your configuration files, enhancing performance by reducing file loading times.
bash php artisan config:cache
Localization Best Practices
For applications supporting multiple languages, manage your localization strings through the resources/lang directory. Aim for consistency by grouping related strings together.
Livewire Best Practices
When working with Livewire components, ensure any configuration specific to these components is kept within relevant config files or environment variables to maintain clarity and ease of adjustments.
By adhering to these best practices, you can streamline your Laravel application’s configuration management, ensuring a more maintainable and scalable project structure.
Enhancing Performance with Caching Strategies in Laravel Applications
Performance issues in Laravel applications often stem from repeated database queries, slow response times, and high server load. Implementing effective caching strategies can significantly mitigate these problems. Some of the most common Laravel API response best practices are:
Common Performance Issues
1. Repeated Database Queries
- Frequent querying of the same data slows down the application.
- Solution: Cache query results to reduce database load.
2. Slow Response Times
- Rendering views and processing complex logic can be time-consuming.
- Solution: Use caching to store pre-rendered views and computational results.
3. High Server Load
- Handling multiple simultaneous requests can strain server resources.
- Solution: Cache static content and frequently accessed data to minimize server processing.
Effective Caching Strategies
1. Query Caching
php $users = Cache::remember(‘users’, 60, function () { return DB::table(‘users’)->get(); });
Stores the result of a database query for 60 minutes.
2. Route Caching
- bash php artisan route:cache
- Combines all route definitions into a single file, reducing the time taken to register routes.
3. View Caching
- bash php artisan view:cache
- Compiles all Blade templates into plain PHP code, speeding up the rendering process.
4. Configuration Caching
- bash php artisan config:cache
- Caches configuration files for quicker access during runtime.
Using these techniques helps alleviate common performance bottlenecks, ensuring a more responsive and efficient Laravel application.
Implementing Robust Logging Mechanisms in Laravel Applications
Effective logging mechanisms are crucial for tracking errors and monitoring application health. By implementing robust logging strategies and Laravel API security best practices, developers can quickly diagnose issues. They can understand application behavior, and improve system reliability.
Importance of Logging in Laravel
- Error Tracking: Logging errors with detailed information helps in identifying and resolving bugs efficiently.
- Monitoring Application Health: Regular logs provide insights into the application’s performance and operational status.
Laravel Logging Best Practices
Use Laravel’s Built-in Logging:
- Laravel provides a powerful logging system based on the Monolog library.
- Customize log channels to direct different types of logs to appropriate destinations (e.g., files, Slack, database).
Log Contextual Information:
- Include contextual data such as user IDs, request URLs, and session info to make logs more informative.
- Use the context parameter in log methods: php Log::info(‘User logged in’, [‘user_id’ => $user->id]);
Leverage Different Log Levels:
- Utilize various log levels (debug, info, notice, warning, error, critical, alert, emergency) to categorize messages appropriately.
Rotate Logs Regularly:
- Prevent log files from becoming too large by rotating them regularly.
- Configure log rotation in the .env file: plaintext LOG_CHANNEL=daily
Monitor Logs Proactively:
- Use monitoring tools that integrate with Laravel’s logging system to get real-time alerts for critical issues.
- Implementing this Laravel architecture best practices helps maintain a healthy and efficient Laravel application, ensuring issues are easily tracked and addressed promptly.
Effective Testing Strategies for Reliable Laravel Applications
Testing is crucial to maintain the reliability and stability of your Laravel applications. Adopting efficient testing strategies ensures that your application behaves as expected under various conditions. Here are some Laravel business logic best practices:
Unit Testing
Unit tests focus on individual components of the application, ensuring each part works correctly in isolation.
- Laravel’s Testing Helpers: Utilize Laravel’s built-in testing tools like PHPUnit and Laravel’s test helpers to write effective unit tests.
- Mocking and Stubbing: Use mocking and stubbing to simulate dependencies and isolate the unit being tested.
Integration Testing
Integration tests examine how different parts of the application work together.
- Database Testing: Leverage Laravel’s in-memory SQLite database or use transactions to ensure database consistency during tests.
- HTTP Tests: Simulate HTTP requests and validate responses using Laravel’s fluent API for making requests.
Functional Testing
Functional tests validate the entire application’s functionality from a user’s perspective.
- Browser Testing: Tools like Laravel Dusk allow for browser-based testing, enabling you to automate user interactions within a real browser environment.
- End-to-End Tests: Combine multiple user flows into comprehensive scenarios to ensure all parts work seamlessly together.
Best Practices for Laravel Testing
Adhering to Laravel unit testing best practices enhances the efficiency and effectiveness of your tests:
- Write Tests Early: Implement tests during development rather than after, facilitating immediate feedback on new code.
- Maintain Test Isolation: Ensure tests do not depend on each other, enabling them to run independently.
- Use Factories and Seeders: Employ Laravel Factories and Seeders to create consistent test data.
Implementing these testing strategies will significantly improve your application’s robustness, leading to a more reliable user experience.
Conclusion
Adopting Laravel development best practices is key to building efficient, secure, and maintainable applications, and Hire Laravel Developer can help you achieve this. By focusing on securing user input, optimizing database interactions, and implementing robust logging mechanisms, Hire Laravel Developer ensures that your applications avoid common pitfalls.
With their expertise, you can stay updated on the latest Laravel deployment, API development, and security best practices. This commitment to high standards will result in reliable, scalable, and user-friendly applications tailored to your business needs.