Simple Tips for Improving Code Quality
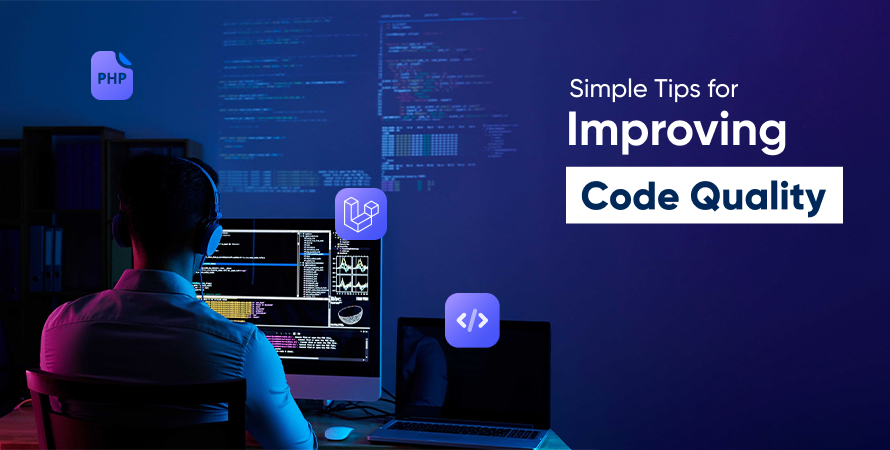
Code quality is a crucial aspect of software development that determines how easily a codebase can be understood, maintained, and extended. High-quality code is not only readable and consistent but also free from bugs and other issues that could compromise the functionality of a project.
Maintaining high code quality is critical for the success of any software project. It ensures that:
- Collaboration among team members is seamless.
- Future modifications to the codebase are easier.
- The software remains reliable and efficient over time.
This article will share simple yet effective tips to help you achieve high code quality in your projects. From adopting coding standards to fostering a culture of continuous improvement, each tip will provide actionable insights into creating robust and maintainable software.
Here, in this blog, you will learn about computer programming best practices. You need to know how to get better at coding to improve code quality.
Adopt Coding Standards
Coding standards play a vital role in ensuring consistency and readability across the codebase. They act as a set of guidelines or coding standards and best practices that developers follow to maintain a uniform coding style.
Key Components of Coding Standards
- Naming Conventions: Define how to name variables, functions, classes, etc., to ensure they are descriptive and consistent.
- File Organization: Establish a structure for organizing files and directories within the project.
- Formatting Rules: Specify indentation, spacing, and other formatting criteria.
Benefits of Adhering to Coding Standards
- Improved Collaboration: Consistent coding styles make it easier for team members to read and understand each other’s code.
- Reduced Errors: Following standardized practices helps catch common mistakes early.
By adhering to these coding standards, development teams can achieve higher levels of collaboration and reduce the likelihood of errors.
Write Automated Tests
Automated tests are essential for maintaining high code quality throughout the development process. They help identify bugs early, ensuring that your quality control code remains robust and reliable.
Types of Automated Tests
Consider implementing various automated tests to cover different aspects of your application:
- Unit Tests: Test individual components or functions to ensure they work as expected.
- Integration Tests: Verify that different pieces of the application work together seamlessly.
- End-to-End Tests: Simulate real user scenarios to test the entire application from start to finish.
Benefits of Automated Testing
- Early Bug Detection: Automated tests catch issues at an early stage, reducing the time and cost associated with fixing them later.
- Increased Confidence: Knowing that your code passes a suite of automated tests boosts confidence when making changes or adding new features.
- Development Efficiency: Automated tests run quickly, allowing for faster feedback loops and more efficient development cycles.
By integrating automated testing into your workflow, you can ensure a more reliable and maintainable codebase, ultimately leading to more successful projects.
Use Version Control Systems like Git
Version control systems are essential tools for managing code changes over time. They allow developers to track modifications, revert to previous versions, and manage multiple branches of development simultaneously.
Benefits of Version Control Systems:
- Change Tracking: Every modification in the codebase is recorded, making it easier to understand the evolution of the project.
- Backup and Recovery: In case of errors or data loss, developers can revert to earlier versions without hassle.
- Branch Management: Multiple features or fixes can be developed in parallel without interfering with the main codebase.
Facilitating Collaboration:
Version control systems excel in collaborative environments. Team members can work on different parts of a project simultaneously, merging their changes seamlessly.
- Conflict Resolution: When two developers make conflicting changes, version control tools help resolve these conflicts efficiently.
- Code Review and Integration: Changes can be reviewed before being integrated into the main branch, ensuring higher code maintainability.
Why Git is Preferred:
Git is one of the most popular and reliable version control tools available today.
- Distributed System: Each developer has a complete copy of the repository, increasing redundancy and reliability.
- Speed and Efficiency: Git handles large projects with ease, offering quick performance even with extensive history.
- Community and Support: A vast community ensures continuous improvement and abundant resources for troubleshooting.
Refactor Code Regularly for Maintainability
Refactoring is the process of restructuring existing code without changing its external behavior. This code best practice is essential for maintaining a clean and manageable codebase. By refactoring, you enhance readability, reduce complexity, and eliminate inefficiencies, which makes future development and debugging tasks easier.
Strategies for Effective Refactoring:
- Eliminate Duplication: Identify and remove redundant code snippets to streamline your codebase.
- Simplify Complex Structures: Break down large functions or classes into smaller, more manageable pieces.
- Improve Naming Conventions: Use meaningful names for variables, functions, and classes to make implementation of code more intuitive.
- Optimize Performance: Refactor inefficient algorithms or data structures for better performance.
Regular refactoring ensures that your code remains in top shape, facilitating ongoing development efforts and making it easier for team members to understand and contribute.
Conduct Code Reviews to Catch Bugs Early On
Conducting regular code reviews within your development team is essential for early bug detection and maintaining high code quality. Code quality standards help ensure that all changes meet the team’s standards and are free of obvious errors before they are merged into the main codebase.
Purpose and Benefits:
- Bug Detection: Regular code reviews can identify potential bugs early in the development process, reducing the cost and effort required to fix them later.
- Knowledge Sharing: These sessions encourage knowledge sharing among team members, leading to a more cohesive and skilled team.
- Consistency: Code reviews help maintain consistency across the codebase by ensuring adherence to coding standards.
Best Practices:
- Set Clear Goals: Define what you aim to achieve with each review, whether it’s catching errors, improving readability, or ensuring adherence to standards.
- Create a Checklist: Having a checklist can streamline the review process and ensure that all essential aspects are covered.
- Limit Review Size: Smaller, more frequent reviews are often more effective than large, infrequent ones.
- Encourage Constructive Feedback: Promote a positive environment where feedback is constructive and aimed at improving the code rather than criticizing the developer.
- Use Code Review Tools: Code quality tools like GitHub’s pull requests or Gerrit can facilitate a smoother review process by providing a structured environment for discussions and revisions.
Implementing these best practices ensures that code steps are productive, fostering an environment of continuous improvement and collaboration within your development team.
Utilize Linters for Consistent Style Guidelines Enforcement
Linters are tools designed to analyze source code for potential errors, including syntax errors, bugs, and deviations from defined style guidelines. By providing real-time feedback as you write code, linters help catch issues before the code is executed.
Key benefits of using linters include:
- Consistency: Linters enforce consistent coding standards across the entire codebase, making it easier to read and maintain.
- Error Prevention: Early detection of syntax errors and potential bugs reduces the chances of encountering runtime issues.
- Productivity: Automated checks save time during code reviews by identifying common issues upfront.
Popular linters like ESLint for JavaScript or Pylint for Python can be integrated into your development environment, ensuring that style guidelines are consistently applied across all projects. This proactive approach to error detection fosters a more reliable and maintainable codebase.
Focus on Clean Code Practices like KISS and DRY Principles
Writing clean, readable, and maintainable code is crucial for long-term project success. Two essential principles for achieving this are the KISS (Keep It Simple Stupid) principle and the DRY (Don’t Repeat Yourself) principle.
1. KISS Principle
The KISS principle emphasizes simplicity in code design. Complex solutions might seem impressive, but they can be hard to understand and maintain. Instead, focus on writing simple, straightforward code that accomplishes its task efficiently. For example, breaking down a large function into smaller, more manageable functions can make your code easier to read and debug.
2. DRY Principle
The DRY principle aims to reduce redundancy in your codebase. Repetition can lead to inconsistencies and make updates more error-prone. By abstracting common functionality into reusable modules or functions, you can ensure that changes need to be made in only one place. This not only enhances maintainability but also reduces the likelihood of introducing bugs.
Adhering to these clean code practices will help you build a more robust and scalable codebase, ultimately leading to higher quality software projects.
Use Smart Code Snippets from Trusted Libraries to Boost Productivity
Using code snippets and frameworks can make your development process more efficient and improve the quality of your work. Instead of starting from scratch every time you need a specific function, you can use existing solutions.
Advantages of Using Trusted Libraries
- Time-Saving: Pre-built code snippets from reputable libraries allow developers to implement complex functionalities quickly.
- Enhanced Quality: Trusted libraries are often well-tested and maintained, ensuring higher quality and reliability.
- Consistency: Utilizing standardized code from well-known sources helps maintain consistency across different parts of the project.
- Focus on Core Features: Frees up time for developers to concentrate on core features rather than boilerplate code.
Example
python import requests
response = requests.get(‘https://api.example.com/data’) data = response.json()
Using the requests library in Python simplifies making HTTP requests compared to writing the entire request logic manually.
By incorporating these practices, you can boost productivity and ensure that your codebase benefits from high-quality, reliable solutions.
Avoid Hard-Coded Values by Utilizing Configuration Files or Constants
Hard-coded values are specific values embedded directly within your application’s logic. This practice can lead to several issues:
- Lack of Flexibility: Making changes requires modifying the source code, re-compiling, and redeploying the application. This is time-consuming and prone to errors.
- Reduced Reusability: Hard-coded values make it difficult to reuse code across different projects or environments.
- Increased Maintenance: Updating hard-coded values scattered throughout the codebase can be tedious and error-prone.
Using configuration files or constants addresses these drawbacks. Configuration files allow you to manage and update settings without altering the source code. Constants provide a central place for defining values that remain consistent throughout your application, improving readability and maintainability.
Example:
python
Using a configuration file
import configparser
config = configparser.ConfigParser() config.read(‘config.ini’)
db_host = config[‘DATABASE’][‘Host’] db_port = config[‘DATABASE’][‘Port’]
Using constants
DATABASE_HOST = ‘localhost’ DATABASE_PORT = 5432
By avoiding hard-coded values, you enhance the flexibility and maintainability of your codebase.
Foster a Culture of Continuous Improvement Within Your Team
Creating a continuous improvement culture within your team is crucial for software reliability and long-term success. Encouraging an environment where team members feel empowered to:
- Regularly review their work: This helps identify potential issues early and fosters a mindset of constant enhancement.
- Seek feedback from peers: Peer reviews provide fresh perspectives and can catch mistakes that might have been overlooked.
- Experiment with new approaches: Innovation often stems from trying out new methodologies or technologies, leading to more efficient and robust solutions.
Conclusion
To maintain high code quality and ensure the long-term success of your projects, it’s essential to implement best practices like adhering to coding standards, writing automated tests, using version control systems, and fostering a culture of continuous improvement.
However, executing these strategies effectively requires experience and expertise. Hiring a Laravel developer from a professional agency ensures your project is in capable hands, with developers who are skilled in creating clean, maintainable, and scalable code. By partnering with the right talent, you can optimize your development process, enhance collaboration, and deliver high-quality software solutions efficiently.