Laravel Validation: Guide for Data Handling
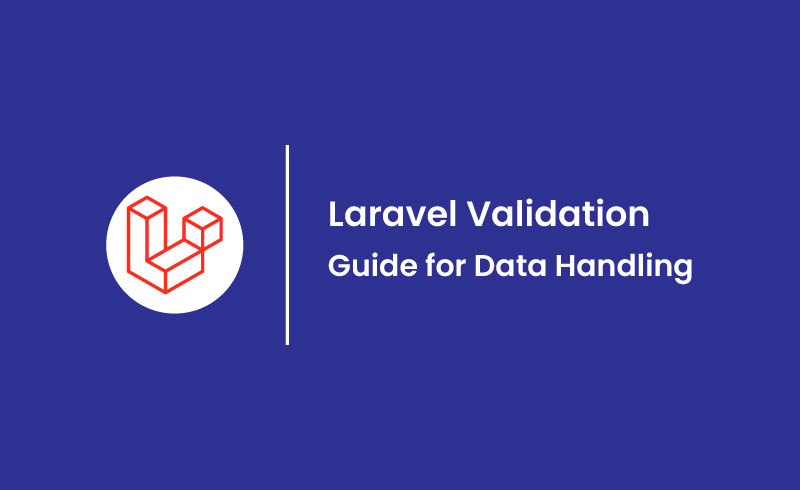
Laravel validation is crucial for web applications, ensuring data integrity and security when dealing with user input. By setting validation rules for incoming data, Laravel guarantees that it meets specific requirements before processing or storing it.
In this guide, we will explore:
- Built-in validation rules.
- Form Request classes.
- Error handling mechanisms.
- Laravel image validation.
Advanced features like conditional rules and Laravel 10 custom validation rules.
Understanding Validation in Laravel
Validation in Laravel is a crucial aspect of maintaining data integrity and security within web applications. It ensures that incoming data adheres to specified criteria before being processed or stored, protecting the application from malicious input and user errors.
Key Built-in Validation Rules
Laravel provides a comprehensive set of built-in validation rules that cater to various data types and scenarios:
- required: Ensures the field is present in the input data.
- email: Validates that the field contains a valid email address.
- unique: Checks if the value is unique in a specified database table.
- max/min: Specifies maximum and minimum length or value for strings and numeric fields.
Example Usage
Here’s a basic example of how these Laravel validation rules can be utilized in a controller method:
php public function store(Request $request) { $validatedData = $request->validate([ ‘name’ => ‘required|max:255′, ’email’ => ‘required|email|unique:users,email’, ‘age’ => ‘required|integer|min:18’, ]);
// Process the validated data
}
This snippet demonstrates validating an incoming request to ensure that name, email, and age fields meet specific criteria before proceeding with any further logic.
Form Request Classes for Encapsulating Validation Logic
FormRequest classes in Laravel data provide an elegant way to encapsulate both authorization and validation logic, leading to cleaner controllers and more maintainable code.
Benefits of Using Form Requests
1. Separation of Concerns
By moving validation logic into its own class, you keep your controllers focused on handling business logic, enhancing readability and maintainability.
2. Reusability
Form Requests can be reused across different controllers, reducing redundancy and ensuring consistent validation rules throughout your application.
3. Authorization Logic
Besides Laravel form request validation, Form Requests also handle authorization checks, allowing you to determine whether the authenticated user is authorized to make the request.
Creating a Custom Form Request Class
- Generate the Form Request Class: bash php artisan make: request StoreUserRequest
- Define Validation Rules: Open the newly created StoreUserRequest class located in the app/Http/Requests directory. Add your validation rules in the rules method: php public function rules() { return [ ‘name’ => ‘required|string|max:255′, ’email’ => ‘required|email|unique:users, email’, ‘password’ => ‘required|string|min:8|confirmed’ ]; }
- Handle Authorization Logic: Define any authorization logic in the authorization method. For instance, if all users are allowed to make this request: php public function authorize() { return true; }
- Use the Form Request in Your Controller: Inject the StoreUserRequest into your controller method to automatically apply validation and authorization checks: php public function store(StoreUserRequest $request) { // Access validated data $validated = $request->validated();
// Handle business logic with validated data… - }
Using FormRequest classes streamlines your code, improves readability, and ensures robust data handling practices.
Client-side vs. Server-side Validation: Pros and Cons
Comparison of Approaches
Client-side validation is performed in the user’s browser before the data is sent to the server. This provides immediate feedback to users, enhancing the user experience by allowing them to correct errors in real-time. Commonly implemented using JavaScript, client-side validation can check for basic input criteria such as required fields or valid email formats.
Server-side validation, on the other hand, occurs on the server after the data has been submitted. This approach ensures that even if a user bypasses client-side checks (e.g., by disabling JavaScript), the data will still be validated securely on the server before any further processing or storage.
Pros and Cons
Client-side Validation:
Pros:
- Immediate feedback for users.
- Reduced server load due to fewer invalid requests.
Cons:
- Can be bypassed by malicious users.
- Relies on client capabilities (e.g., JavaScript enabled).
Server-side Validation:
Pros:
- Essential for security; cannot be bypassed by manipulating client-side code.
- Centralized validation logic ensures consistency across different clients.
Cons:
- Potentially slower feedback as Laravel required without a round trip to the server.
- Higher server load due to processing all validation checks.
Importance of Robust Server-side Validation
Robust server-side validation is crucial for maintaining data integrity and securing your application against attacks such as SQL injection and XSS. While client-side validation improves user experience, it should always be complemented with comprehensive server-side checks to ensure that only valid and safe data is processed. Laravel’s built-in validation features make it seamless to implement strong server-side validation rules, providing a solid foundation for secure web applications.
Error Handling in Laravel Validation: A Seamless User Experience
Laravel provides an intuitive way to manage validation errors during form submissions. When validation fails, the framework automatically handles error messages and redirects users back to the previous page.
Key Mechanisms
1. Automatic Redirection
Upon a failed validation, Laravel redirects users back to the previous page with all input data and error messages stored in the session. This ensures that users don’t lose their input and can quickly correct their mistakes.
2. Session Storage for Error Messages
Error messages are stored in the session, allowing developers to easily display them in views. These messages can be accessed using $errors variable, making it straightforward to inform users about what went wrong.
3. User-friendly Feedback
By leveraging Blade templates, you can display meaningful error messages in forms. For example:
php @if ($errors->any()) @foreach ($errors->all() as $error) {{ $error }} @endforeach @endif
This approach enhances user experience by providing clear and actionable feedback, encouraging timely corrections and smoother interactions with your application.
Customizing Error Messages for Better User Feedback
Creating more intuitive and informative error messages in Laravel validation enhances user experience significantly. Laravel allows developers to customize these messages easily:
1. Custom Message Definitions
Define custom messages directly in the validation array using the messages method within your Form Request class.
php public function messages() { return [ ’email.required’ => ‘We need to know your email address!’, ‘password.min’ => ‘The password must be at least :min characters long.’, ]; }
2. Attribute Customization
Customize attribute names for better readability using the attributes method.
php public function attributes() { return [ ’email’ => ’email address’, ‘password’ => ‘account password’, ]; }
These strategies help in providing user-friendly responses, making error handling more effective and comprehensible.
Conclusion
Leveraging Laravel’s request validation system is crucial for ensuring secure and reliable data handling across your web applications. From built-in validation rules to advanced customizations, Laravel provides a comprehensive toolkit to enhance application security and maintain data integrity.
To fully take advantage of these powerful features, it’s wise to hire a Laravel developer from an experienced agency. Their expertise will ensure proper implementation of robust server-side validation, safeguarding your application from malicious inputs while improving user experience. By partnering with skilled professionals, you can streamline your development process and build secure, user-friendly applications confidently.