Laravel & React: The Perfect Pair for Modern Web Development
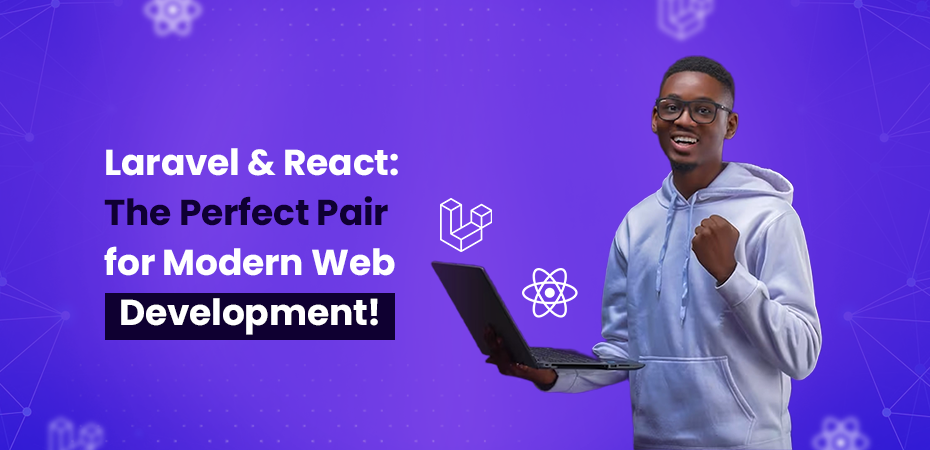
Are you ready to supercharge your web development journey? Let’s dive into the dynamic duo that’s changing the game for modern web applications: Laravel and React JS.
Think of Laravel as your backend powerhouse – a PHP framework that handles server-side operations with elegance and efficiency. Now, add React to the mix – Facebook’s innovative JavaScript library that creates stunning, interactive user interfaces. Together, they form an unstoppable combination.
Why should you care about this pairing? Laravel MVC with React provides a seamless solution for building modern, dynamic web applications by combining Laravel’s robust MVC architecture with React’s interactive user interfaces. Here’s what makes it special:
- Lightning-fast performance: Laravel’s efficient backend processing + React’s Virtual DOM
- Seamless user experiences: React’s component-based architecture creates fluid interfaces
- Rock-solid security: Laravel’s built-in protection against common web vulnerabilities
One of the best ways to create new Laravel API projects is by combining the power of Laravel’s backend architecture with React’s dynamic frontend capabilities. Ready to explore how these technologies can transform your web development process? Let’s proceed.
Understanding Laravel and React
Laravel: Your Backend Powerhouse
Laravel is the most advanced framework for backend development in PHP. It serves as the core of your application, handling all the critical tasks behind the scenes.
Key Laravel Features:
- Eloquent ORM – Write database queries using simple PHP syntax instead of complex SQL
- Blade Templating – Create dynamic web pages with an expressive, yet lightweight syntax
- Artisan CLI – Automate common tasks with built-in command-line tools
- Database Migrations – Version control your database structure with simple PHP files
- Built-in Authentication – Secure user management right out of the box
Laravel’s design makes complicated tasks seem easy. For example, instead of writing lengthy SQL queries to get user data, you can use Eloquent to simply say User::where(‘active’, true)->get(). Similarly, when creating a view, you can use Blade’s clean syntax to write @foreach($users as $user) instead of traditional PHP loops.
React: Crafting Interactive Frontends
Using react framework changes the way we build user interfaces. Developed by Facebook, this JavaScript library breaks down complex UIs into smaller, reusable components.
React’s Core Strengths:
- Component-Based Architecture – Build UIs like Lego blocks, piece by piece
- Virtual DOM – Update only what needs changing, keeping performance high
- JSX Syntax – Write HTML-like code directly in JavaScript
- State Management – Track and update data efficiently across components
- Rich Ecosystem – Access thousands of pre-built components and tools
React’s PHP framework is particularly good at building Single Page Applications (SPAs). Unlike traditional websites that load entire pages, SPAs only refresh specific parts of the site instantly. A perfect example of this is how Facebook updates your news feed without requiring a page reload – that’s React working behind the scenes.
jsx function Welcome({ name }) { return
Hello, {name}!
; }
The above code snippet demonstrates a basic React component. It showcases how effortlessly reusable UI elements can be crafted. With this approach, you have the flexibility to display various names using a single component without any need for code duplication.
Benefits of Using Laravel with React
The combination of Laravel and React creates a powerful synergy that elevates web development to new heights. Let’s explore the key advantages of this dynamic duo.
Separation of Concerns
The Laravel-React stack enables a clean separation between backend logic and frontend interfaces. This architectural approach brings several advantages:
- Maintainable Codebase: Backend developers can focus on API development and database operations while frontend developers work independently on UI components
- Easier Testing: Isolated components allow for more focused and effective testing strategies
- Simplified Debugging: Issues can be pinpointed and resolved quickly within their specific domain
- Independent Scaling: Each layer can be scaled according to specific needs without affecting the other
Enhanced Developer Experience
The development workflow becomes streamlined with React and Laravel’s built-in tools:
Laravel Productivity Boosters
- Artisan CLI for automated task handling
- Built-in authentication scaffolding
- Database migration system
- Automated testing tools
React Development Benefits
- Hot module replacement for instant feedback
- Reusable component library
- Rich ecosystem of UI components
- Developer tools for debugging
Scalability and Security
The Laravel(PHP)-React(JS) stack is built to handle growth and protect your applications:
Scalability Features
- Laravel’s Queue System: Handles background jobs efficiently
- React’s Virtual DOM: Optimizes rendering performance
- API Resource Management: Efficient data handling between frontend and backend
- Lazy Loading: Improved load times for large applications
Security Measures
- CSRF Protection: Automatic security token generation
- SQL Injection Prevention: Query builder and ORM security
- XSS Protection: React’s built-in HTML escaping
- Authentication System: Secure user management
- API Authentication: Token-based security for data access
The combination of these features creates a robust foundation for building complex web apps. Laravel’s backend strength paired with React’s frontend capabilities enables developers to create performant, secure, and scalable solutions that meet modern web development demands.
Laravel is great for backend development, but many developers choose to use Laravel with Next.js for full web applications. Next.js, which builds on React, offers powerful features like server-side rendering (SSR) and static site generation (SSG). This makes it a great combination with Laravel, as it provides both a flexible backend and a fast, SEO-friendly frontend.
Step-by-Step Guide to Integrate Laravel with React
Here’s a practical guide to set up your React JS-Laravel project. Follow these steps for a smooth integration:
1. Set Up Your Laravel Project
In Laravel, get started with creating a new (Laravel) project and navigate to the project directory:
composer create-project laravel/laravel your-project-name
cd your-project-name
After installation, verify your setup by running:
php artisan serve
This should start the Laravel development server.
2. Install React in Your Project
Laravel offers built-in scaffolding for React via Laravel UI. To install it, run:
composer require laravel/ui
php artisan ui react
npm install
3. Manage NPM Dependencies
Install essential packages for React development:
npm install @babel/preset-react
npm install react-router-dom
npm install axios
Then, configure webpack.mix.js to compile the React code properly. Add the following lines:
mix.js(‘resources/js/app.js’, ‘public/js’)
.react()
.postCss(‘resources/css/app.css’, ‘public/css’);
4. Create React Components
Inside resources/js, create a components directory:
mkdir resources/js/components
Then, create a simple component, for example, Example.js:
// resources/js/components/Example.js
import React from ‘react’;
function Example() {
return (
<div>
<h1>Hello, React!</h1>
</div>
);
}
export default Example;
5. Render Components Using Blade Templates
In your Blade view file, you can now render your React components. For example, in a Blade template (resources/views/welcome.blade.php), include your React component like so:
@extends(‘layouts.app’)
@section(‘content’)
<div id=”app”></div>
<script src=”{{ mix(‘js/app.js’) }}”></script>
@endsection
This will allow Laravel to handle routing and rendering while React controls the front-end behavior.
6. API Integration
To make your React components dynamic, you can integrate them with Laravel’s backend via APIs. For this, create routes, controllers, and API endpoints in Laravel to serve data, which your React components can fetch using Axios or other methods.
For example, in Laravel, you can create a controller:
php artisan make:controller ApiController
In the controller, create an API endpoint:
public function fetchData() {
return response()->json([‘message’ => ‘Hello from Laravel’]);
}
And define a route:
Route::get(‘/api/data’, [ApiController::class, ‘fetchData’]);
In your React component, use Axios to fetch the data:
import React, { useEffect, useState } from ‘react’;
import axios from ‘axios’;
function Example() {
const [message, setMessage] = useState(”);
useEffect(() => {
axios.get(‘/api/data’)
.then(response => setMessage(response.data.message))
.catch(error => console.log(error));
}, []);
return (
<div>
<h1>{message}</h1>
</div>
);
}
export default Example;
This step-by-step guide outlines how to integrate Laravel with React. With React handling the front-end and Laravel providing the backend API, you can build powerful and dynamic web applications.
Conclusion
Laravel and React are excellent choices for modern web development. This powerful combination brings together Laravel’s robust backend capabilities with React’s dynamic frontend features to create exceptional web applications.
For developers aiming at creating useful Laravel apps, integrating React JS (doc) provides the perfect frontend solution. The integration process, while requiring careful planning, rewards developers with a versatile toolkit for crafting sophisticated web applications. Laravel’s elegant syntax paired with React’s efficient DOM manipulation creates a development environment where innovation thrives.
Ready to elevate your web development projects? Our team of dedicated Laravel developers specializes in creating cutting-edge applications that leverage the full potential of Laravel and React. Contact us to discuss your next project and discover how we can help bring your vision to life.
Frequently Asked Questions (FAQs)
Yes! Laravel works great with other frontend frameworks like Vue.js or can be used with its Blade templating system alone.
Yes, you’ll need basic knowledge of PHP for Laravel backend development and JavaScript for React frontend work.
While it’s powerful for large applications, this combo might be overkill for simple websites. Consider your project requirements carefully.
Both have moderate learning curves. Prior experience with PHP helps with Laravel, while JavaScript knowledge makes React easier to grasp.
Yes, though VPS or cloud hosting platforms like AWS or DigitalOcean are recommended for better performance and control.ct!