Laravel Nova: A Comprehensive Guide
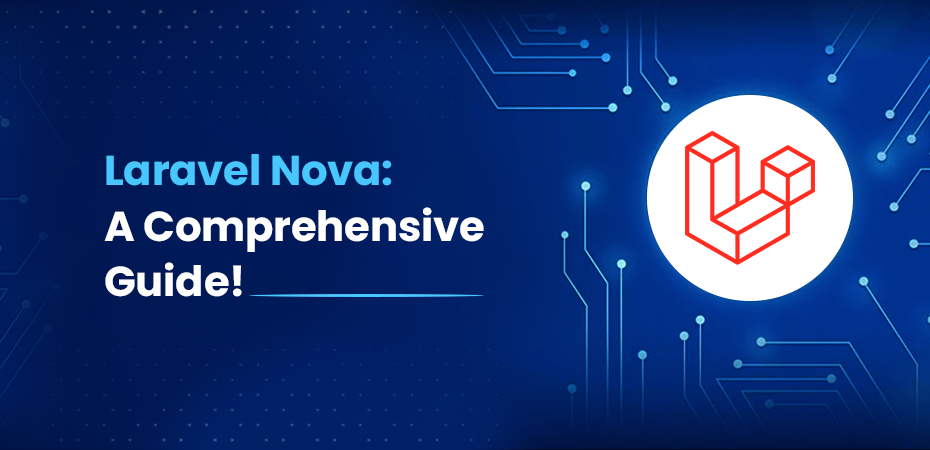
Laravel Nova is a beautifully designed admin panel for Laravel applications, offering a powerful and flexible Laravel dashboard that allows you to manage your data with ease. It integrates seamlessly with Laravel’s backend, providing an intuitive interface to work with your application’s database without writing complex code. Whether you’re building a small Laravel website or a large-scale Laravel ecommerce project, Nova can simplify your workflow and improve productivity. Let us define Nova first to have a better understanding.
Nova Definition: It isn’t just about creating an admin panel—it’s a tool that enhances your application’s usability. As Laravel’s flagship admin solution, it allows developers to quickly define resources, manage data, and implement complex relationships using a visual interface.
For developers who love Laravel’s ecosystem, it provides an integrated dashboard HTML template that simplifies building scalable applications. Thus, in the context of Laravel, Nova is defined as is an administration panel for Laravel applications that provides a beautifully designed interface for managing resources and data.
Why Choose Nova?
Many other admin panels exist, but Laravel Nova stands out because of its deep integration with Laravel. Whether you’re using it for simple CRUD (Create, Read, Update, Delete) operations or integrating more advanced features like Spatie Permissions, Nova can handle it all. Additionally, with features like resource management, custom fields, and powerful filters, you can create fully-customizable dashboards and admin panels that fit your application’s needs.
Key Features of Laravel Nova
Laravel Nova is packed with a wide range of features that simplify the creation and management of admin panels for your web applications. Some of its most compelling features include:
- Elegant Admin Panel: Nova’s UI is sleek, clean, and highly intuitive, making it one of the most appealing admin dashboards available for Laravel applications. The polished design doesn’t sacrifice functionality for aesthetics. This combination of form and function makes it a go-to for developers who need a modern backend interface.
- Resource Management: One of Nova’s core strengths is its handling of resources. In Nova, a resource is a representation of a model in your database. For instance, a User model will have a corresponding UserResource in Nova. This resource-driven architecture allows developers to manage, create, and update data seamlessly. Nova’s resource relationships feature lets you define complex data structures like HasMany, BelongsTo, and ManyToMany relationships right within the dashboard.
- Powerful Search and Filtering: Nova includes a built-in global search bar that lets you search across all resources and models in your application. This feature allows users to quickly find and manage data without sifting through individual resources. You can also define filters for resources, so your users can further refine their searches. This advanced search functionality is enhanced when paired with Laravel Scout, which allows you to integrate third-party search platforms like Algolia for lightning-fast queries.
- Custom Fields and Actions: Out of the box, Nova provides a wide range of field types, such as text, boolean, date, image, file upload, and more. But one of Nova’s standout features is its ability to create custom fields. If your application requires a custom input or specific action, you can easily build it using Nova’s extensible field architecture. In addition, custom actions allow you to define tasks that can be performed on one or more resources. For example, sending emails or exporting data can be triggered directly from the dashboard, saving you time and effort.
- Authorization and Policies: Nova leverages Laravel’s robust authorization features to control access to resources, actions, and fields. For example, by using Laravel’s built-in policies, you can specify who can view, update, or delete specific resources. This is especially useful for applications with role-based access controls. Coupled with Spatie Permissions, you can implement fine-grained control over user roles and actions, making Nova a secure choice for admin panels with sensitive data.
- Custom Dashboards and Metrics: Nova offers a range of customization options, including dashboards that can display various metrics about your application’s performance. These custom metrics can be built using cards, which can include visualizations such as charts, tables, and graphs. This feature is perfect for developers who need to track KPIs or monitor critical data trends within the application.
- Modular and Extendable: Nova is not just limited to what comes out of the box. It supports third-party packages and integrations, making it an ideal solution for complex projects. Nova Lib. like Volt Admin Laravel Git and Hope UI Laravel Git can be integrated to add more functionality or enhance the UI, allowing you to build more sophisticated admin systems.
Installation and Setup
Getting started with Laravel Nova is straightforward, but there are a few important steps to ensure smooth setup:
Step 1: Prerequisites: Before diving into Nova, you need to make sure your environment is ready. First, you should have a working Laravel installation and a licensed copy of Nova. Laravel Nova is a paid tool, and you’ll need to purchase a license from nova.com. Also, ensure that your PHP and Composer versions meet the minimum requirements set by Laravel.
Step 2: Installing Nova via Composer: Once you have your license, you can install Nova using Composer. Run the following command in your terminal:
composer require laravel/nova
This command downloads Nova and installs it as part of your Laravel application.
Step 3: Configuring Nova: After installing Nova, you need to configure it. You can publish Nova’s configuration files and assets using the following Artisan command:
php artisan nova:install
This will register the necessary Nova service provider in your application.
Step 4: Setting Up Resources: With Nova installed, the next step is to create your resources. Each resource in Nova corresponds to a model in your application. For example, if you have a User model, you can create a corresponding UserResource using the command:
php artisan nova:resource User
This generates the resource class and sets up a framework for managing the User model from the Nova dashboard. You can now define fields, relationships, and actions for this resource.
Step 5: Configure Routes: Nova comes with pre-configured routes, making it easy to access the admin panel. Simply navigate to /nova in your browser to access your Nova dashboard. You can customize the route if needed, especially in cases where security is a concern, and you want to obscure the default admin URL.
Step 6: Authentication: Nova uses Laravel’s built-in authentication system. When you try to access Nova for the first time, Nova asks for a password in managed account. You’ll need to ensure proper user authentication is in place to manage the dashboard.
Managing Resources in Nova
Managing resources in Laravel Nova is both intuitive and powerful. Each Nova resource corresponds to a model in your application, which could represent users, products, orders, etc.
- Defining Resources and Relationships: Nova app makes it easy to manage Eloquent relationships, whether it’s a simple Has Many or complex Belongs To Many relationship.
- Customizing Fields: Nova management offers many built-in fields like text, images, and files. If you need something custom, like a special dropdown or file upload, you can define your own field types. Custom fields allow you to tailor your Nova instance to meet the exact needs of your application.
- Handling Validations: Nova also integrates with Laravel’s validation system, allowing you to apply complex validation rules to your fields, ensuring data integrity in your application.
Customization and Flexibility
One of the most powerful aspects of Laravel Nova is its ability to be customized. From custom fields and actions to custom dashboards, you can mold Nova to fit your unique application needs.
- Custom Fields and Actions: You can create actions that are triggered by the user in the Nova dashboard. For example, creating an action that sends an email to users or updates multiple records at once.
- Custom Dashboards: Nova supports fully-customizable dashboards, where you can include metrics, charts, and even embed third-party tools like Nova Library Database or Nova Application.
- Nova Cards and Lenses: Cards in Nova allow you to display additional information on your dashboard. Lenses offer more advanced ways to query and display your data.
Authorization and Permissions
Nova uses Laravel’s built-in authorization system to manage access to resources. By leveraging Laravel Policies, you can define who can view, create, or update specific resources.
- Role Management: Integrating with packages like Spatie Permissions allows you to create fine-grained control over user roles and permissions, ensuring only authorized users can access certain parts of your Nova admin panel.
- Protecting Resources: By configuring policies, you can control access down to individual fields or actions, making Nova an ideal choice for applications requiring robust security.
Best Practices for Building with Laravel Nova
Building with Laravel Nova can become complex as your application grows. Following these best practices will help keep your project maintainable and efficient:
- Organize Your Resources: One of the easiest ways to maintain clarity within your Nova dashboard is to keep your resources organized. For larger applications, consider grouping related resources. This not only makes navigation easier but also enhances user experience by allowing admins to find what they need more quickly.
- Leverage Custom Filters and Lenses: If your application handles large datasets, make use of custom filters and Nova Lenses. Filters allow you to narrow down data based on specific criteria, while Lenses provide an alternative way to display resource data. These tools can enhance performance and user experience by making large amounts of data more manageable.
- Optimize Performance: Admin dashboards tend to become data-heavy, which can affect performance. Use Laravel’s built-in tools like Telescope to monitor database queries, page load times, and other performance metrics. Nova allows you to optimize queries using tools like Laravel Scout or by customizing Eloquent queries in your resources. Always use Get all query get Laravel with careful consideration to avoid pulling large amounts of unnecessary data.
- Secure Your Nova Dashboard: Security should always be a priority, especially when building an admin panel. Implement Spatie Permissions to control access, and use policies to ensure that users only see data they are authorized to view. Always keep Nova’s security patches and Laravel core up to date to avoid vulnerabilities.
- Maintain a Clean Codebase: As you extend Nova with custom fields, actions, and tools, your codebase can become cluttered. Ensure that your custom logic is well-organized, and where possible, break it into smaller reusable components. For example, if you’re integrating the Hope UI Laravel Git or Volt Admin Laravel Git libraries, ensure they are modular and easily maintainable.
- Plan for Scalability: If you anticipate your application growing significantly, consider how Nova will scale with it. Large applications with complex relationships can slow down if not optimized. Using Nova Catalog Architecture can help you organize your resources for larger data sets.
Pros and Cons of Using Laravel Nova
Pros
- Deep Integration with Laravel: One of Nova’s biggest advantages is its seamless integration with Laravel. It uses the same Eloquent models, policies, and validation systems that you’re already familiar with, meaning you don’t have to learn a completely new framework.
- Customizability: Nova is highly customizable, allowing developers to create custom fields, actions, and even dashboards. This means that whether you’re building a simple admin panel or a complex data management system, Nova can be adapted to your needs. The ability to extend Nova with third-party packages, like Spatie Permissions, makes it versatile for different applications.
- Elegant and Intuitive UI: Nova’s design is clean, modern, and intuitive. Admin users, even those without technical backgrounds, will find it easy to navigate and manage data. The Nova Logo and branding also add to its polished, professional appearance.
- Time-Saving: Developers can save a lot of time using Nova as opposed to building an admin panel from scratch. With built-in support for CRUD operations, data relationships, and search functionality, Nova handles a lot of the heavy lifting.
- Active Ecosystem: Nova has a growing community and many third-party packages, allowing developers to extend its functionality in new and creative ways. Examples like Volt Admin Laravel Git and Hope UI Laravel Git can be integrated seamlessly.
Cons
- Cost: Unlike most open-source Laravel tools, Nova requires a paid license, which could be a limiting factor for developers or small businesses with a tight budget.
- Not Suitable for Every Use Case: While Nova is great for many types of applications, it may not be ideal for applications that require extremely custom admin features or those that don’t follow Laravel’s architecture closely. For very lightweight admin panels or applications that already have unique dashboard requirements, building a custom solution might be more efficient than using Nova.
- Learning Curve for Customizations: While Nova is easy to use for basic CRUD applications, getting into custom fields, lenses, and advanced relationships may take some time, especially for developers new to Laravel or Nova. Large-scale projects like Nova Course Catalog or Nova Masters might require extensive customization, which can add to the development timeline.
- Performance Issues with Large Data Sets: Nova can become slow when managing large datasets, especially if not optimized. If your application involves massive data sets like Nova Database Library, special attention needs to be given to optimizing queries and minimizing resource load.
If you’re working on projects like Nova Catalog Architecture or large-scale applications, Nova can simplify the process but might have some limitations in highly specific scenarios.
Conclusion
Laravel Nova is a powerful admin panel that integrates seamlessly with Laravel, providing developers with the tools needed to manage complex data relationships, user permissions, and custom dashboards. Whether you’re using My Nova for your first Laravel application or integrating it with larger systems, Nova can simplify backend management while offering deep customization options.
If you’re ready to try Laravel Nova, you can purchase a license from nova.com, download it, and start building powerful admin panels today.
Nova makes building robust Laravel applications easier, more elegant, and highly scalable.