Domain-Driven Design – A Comprehensive Guide
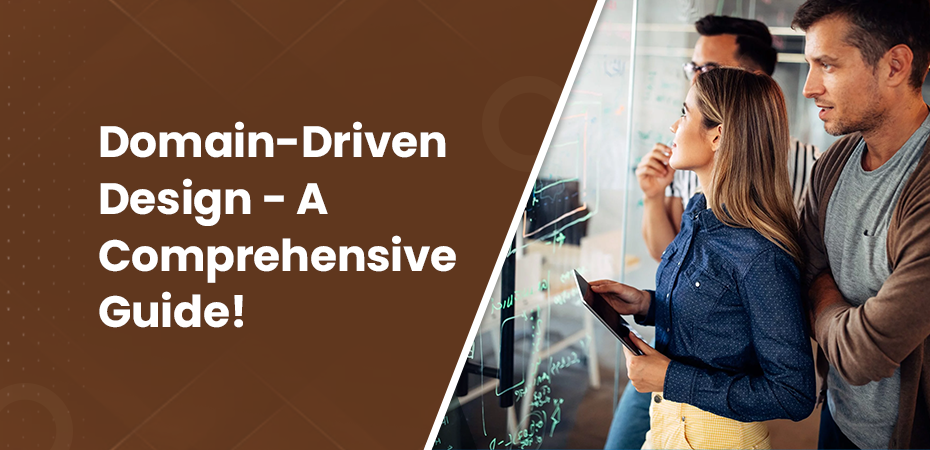
In today’s fast-paced world of software development, complex systems often require more than just technical expertise—they demand a deep understanding of the business domain to ensure that software solutions align with real-world needs. Enter DDD, a powerful approach to managing complexity in software design by emphasizing a close collaboration between technical and business experts. But before moving further, let us understand what DDD stands for?
- D – Domain
- D – Driven
- D – Design
Originally popularized by Eric Evans in his book “Domain-Driven Design: Tackling Complexity in the Heart of Software” (2003), DDD focuses on creating software that reflects the core business rules, processes, and needs. This guide will help you understand DDD meaning, its key principles, common examples, and how to implement it effectively.
What is Domain-Driven Design?
Domain-Driven Design (DDD) is a programming software design methodology that focuses on understanding the business domain in which the software will operate. Instead of just focusing on the technical aspects, DDD puts the domain (the problem space) at the center of software development, ensuring the business logic drives the solution.
Key Objectives of DDD (Domain Driven Design)
- Close collaboration between domain experts and developers to create models that represent the business rules and processes.
- Alignment of software with business needs by constantly evolving the design around the domain.
- Reducing complexity by breaking large systems into smaller, manageable pieces (subdomains and bounded contexts).
The primary goal of DDD design is to ensure the software development process mirrors the real-world domain, leading to more accurate, maintainable, and scalable software.
Domain Driven Design Example
To further understand DDD, it’s helpful to look at real-world example that illustrate Domain Driven Design’s architecture and practical application.
- E-commerce System
Consider an online shopping platform. Here’s how DDD would structure this domain:
Entities
Customer (has a unique identity)
Order (tracked uniquely by an ID)
Value Objects
Address (can be part of the customer entity but doesn’t need a unique ID)
Product Price (represented by a value object to ensure consistency)
Bounded Contexts
Customer Management (handles customer data and orders)
Inventory Management (handles stock levels and products)
Each bounded context would have its own models and logic, ensuring that changes in one part of the system don’t negatively impact another.
Domain-Driven Design Principles
DDD is built on a set of foundational principles that guide its implementation:
Ubiquitous Language
In DDD, language plays a crucial role. Developers and domain experts must share a Ubiquitous Language—a shared, consistent vocabulary that is used throughout the project. This reduces misunderstandings and ensures that everyone is on the same page.
Bounded Contexts
Software systems often encompass multiple subdomains with different rules. To avoid confusion and overlap, DDD advocates breaking the system into Bounded Contexts, each representing a distinct part of the overall system with its own models and logic.
Entities and Value Objects
Entities are objects that have a unique identity within a domain, such as a customer or an order. Value Objects, on the other hand, represent attributes or descriptions but do not have a unique identity. In DDD, distinguishing between these helps in building a robust domain model.
Aggregates
An Aggregate is a collection of entities and value objects that are treated as a single unit. Aggregates are used to enforce business rules, ensuring that changes to the system are consistent. Each aggregate has an Aggregate Root, which is the main entity responsible for maintaining consistency across the aggregate.
Repositories
To manage aggregates, DDD uses Repositories. These are interfaces that provide methods for retrieving, adding, or modifying aggregates from a data store, without exposing the complexities of the underlying infrastructure.
Domain Events
Domain Events capture things that have happened in the domain that are of significance. For example, in an e-commerce system, “Order Placed” or “Product Out of Stock” would be domain events. These events can trigger reactions elsewhere in the system.
Key Concepts and Building Blocks of DDD
Domain
The domain is the core area of focus. It represents the problem space you’re working to address. Domains can be divided into:
- Core Domains: The most critical to the business, where competitive advantage lies.
- Supporting Domains: Necessary but secondary to the core domain.
- Generic Domains: Generic processes that don’t offer a competitive edge but are required, such as logging.
Subdomains
Breaking down the domain into Subdomains allows teams to focus on different areas of the system. Each subdomain typically becomes a bounded context, which provides a clear boundary for modeling the system’s behavior.
Entities and Value Objects
Entities are objects with a unique identifier, while Value Objects have no identity but represent values critical to the domain. For example, a customer is an entity, but their address could be a value object.
Aggregates and Aggregate Roots
Aggregates are clusters of objects bound together by a root entity called the Aggregate Root. They ensure consistency in the domain model by controlling how related entities and value objects interact.
Tactical Design Patterns in DDD
Bounded Contexts
A bounded context is a boundary around a subdomain where a particular model is defined and applicable. Each bounded context contains a Ubiquitous Language specific to that subdomain, preventing the leakage of business logic into other areas of the system.
Context Mapping Techniques
In more complex systems, bounded contexts may need to interact. Context Mapping Patterns like Shared Kernel, Customer-Supplier, and Anti-Corruption Layer help manage relationships between these contexts, ensuring that each stays true to its unique domain model.
Ubiquitous Language
The language of the business domain should be consistently used across code, documentation, and communication to maintain clarity and avoid misunderstandings. This makes sure that domain models reflect real-world processes.
Strategic Design in DDD
Event Storming
Event Storming is a collaborative workshop where domain experts and developers map out domain events. This visual approach helps in quickly identifying key events and modeling the flow of the system.
Domain Events
Domain events reflect meaningful changes within the system. For example, when a customer places an order, that event triggers processes like inventory checks, payment processing, and shipping notifications.
Event-Driven Architecture
In an Event-Driven Architecture, systems react to domain events, making the system highly decoupled and responsive to real-time changes. This architecture is often used with Event Sourcing, where state changes are stored as a series of events rather than as direct updates to data.
Benefits and Challenges of Implementing DDD
Benefits:
One of the biggest advantages of Domain-Driven Design (DDD) is its ability to bridge the gap between developers and business stakeholders. By encouraging close collaboration and the use of a Ubiquitous Language, everyone involved in the project can communicate effectively, reducing misunderstandings and ensuring the software closely reflects the real-world business domain.
DDD also allows for better system design, as it encourages focusing on the most critical parts of the business, known as core domains, which drive competitive advantage. This ensures that the design of domains evolves with changing business needs, making the software more adaptable over time.
Another major benefit is scalability. By breaking down large systems into bounded contexts and subdomains, DDD allows for smaller, more manageable pieces that can be worked on independently, making it easier to scale complex systems. This modular approach also makes it easier to maintain and extend the system in the future.
Challenges:
Despite its benefits, DDD is not without challenges. One of the primary obstacles is the initial complexity. Establishing the right bounded contexts, defining entities, value objects, and aggregates can be overwhelming, especially for teams unfamiliar with DDD principles. Additionally, DDD can be overkill for smaller projects where the domain is straightforward and doesn’t require intricate modeling. It also requires a significant time investment upfront to collaborate with domain experts and build the domain model correctly, which can be a challenge in fast-paced environments. Lastly, there’s a learning curve involved—teams need to understand the key concepts and how to apply them effectively, which can take time.
Use Cases of Domain-Driven Design
Domain-Driven Design shines particularly well in complex software systems where deep business logic and constant evolution are at play. Here are some common use cases for DDD:
E-commerce Platforms
E-commerce systems are a great fit for DDD because they involve multiple business processes, from managing products and inventory to handling customer orders and payments. DDD helps break down these complexities into smaller, well-defined domains. For example, a Customer Management context might focus on user profiles and order history, while an Inventory Management context could handle stock levels and pricing. By separating these concerns, DDD allows each part of the system to evolve independently.
Financial Services and Banking Applications
In industries like banking, the complexity of domain logic is immense, with requirements for security, transactions, compliance, and customer management. A Loan Processing context, for instance, would handle loan applications, interest calculations, and repayments, while a Customer Accounts context would manage deposits and withdrawals. DDD ensures that the financial rules and policies are encapsulated within each domain, improving both clarity and compliance.
Healthcare Systems
Healthcare systems involve the management of patient records, treatments, insurance claims, and more. By applying DDD, each of these areas can be modeled as a separate bounded context. For instance, a Patient Management context can focus on handling patient data and appointments, while a Billing context manages payments and insurance claims, ensuring that each area is managed consistently and can evolve independently.
Large-Scale Enterprise Applications
Organizations with sprawling systems—such as customer relationship management (CRM) tools, supply chain management, or enterprise resource planning (ERP) systems—can use DDD to break down their massive, interconnected processes into smaller, manageable modules. By focusing on individual domains, businesses can ensure that their software remains aligned with evolving business strategies.
These use cases illustrate how Domain-Driven Design is particularly suited for complex, evolving systems where business rules and domain logic are critical to success. By dividing a large system into manageable parts, DDD makes it easier to implement, maintain, and scale the system as the business grows.
Conclusion
Domain-Driven Design offers a powerful approach to handling complex software systems by focusing on collaboration, domain models, and aligning software with real-world business needs.
Though it comes with challenges, its benefits make it an invaluable methodology for creating scalable, maintainable, and relevant systems. With a solid understanding of DDD principles, tactical patterns, and real-world examples, teams can take advantage of DDD to deliver better software that mirrors business domains.