API Rate Limiting in Laravel: Everything You Need to Know
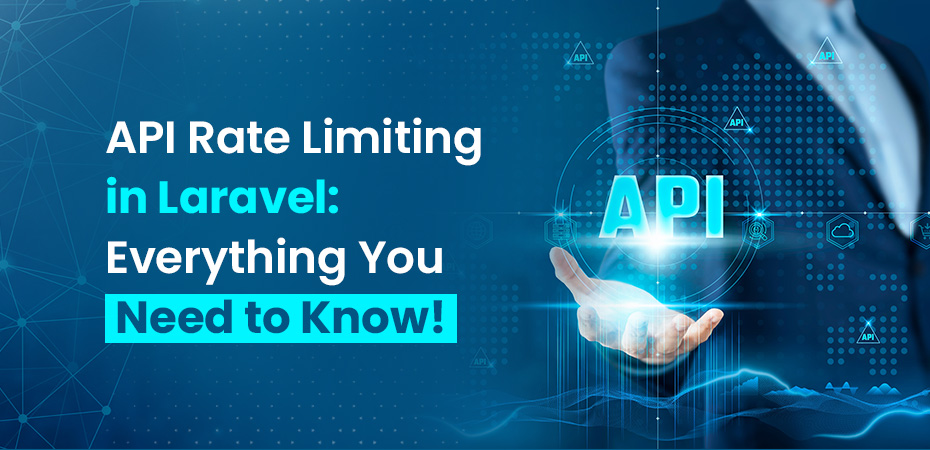
Ever wondered how major web applications handle millions of API requests without breaking a sweat? The secret lies in API rate limiting – a crucial feature that’s built right into Laravel’s core.
Think of API limit request threshold as a traffic controller for your web application. It prevents your servers from being overwhelmed by managing how many requests a user can make within a specific timeframe. This protection is essential for:
- Maintaining optimal application performance
- Preventing abuse and unauthorized access
- Ensuring fair resource distribution among users
- Protecting against DDoS attacks
“Rate limit exceed” means a user or system has made more requests than allowed within a specific time frame, surpassing the set limit. This results in the system blocking or slowing down further requests until the limit resets.
Laravel makes implementing rate limiting a breeze with its powerful built-in features. You’ll find everything from simple IP-based limits to advanced user-role settings that are ready to use right away.In this guide, we’ll dive deep into Laravel’s rate limiting capabilities.
You’ll learn how to set up basic limits, create custom configurations, and implement advanced strategies to protect your APIs. Whether you’re building a small application or scaling to enterprise level, these techniques will help you maintain control over your API traffic.
Understanding API Rate Limiting
To define rate limiting, think of it as a safeguard to prevent overuse of your API by enforcing restrictions on how frequently a user or client can make requests.
It works like a traffic cop for your website, controlling how many requests a user or client can make in a set amount of time. It’s like setting a speed limit for your API!
Here’s what API rate limiting helps you achieve:
- Resource Protection: Prevents web server overload by limiting the number of incoming requests
- Fair Usage: Ensures equal resource distribution among all users
- Cost Control: Reduces infrastructure expenses by preventing excessive API consumption
Rate limiting becomes crucial in defending against several common threats:
- Brute Force Attacks: Blocks repeated login attempts by limiting authentication requests
- DDoS Attacks: Mitigates distributed denial-of-service attacks by capping requests from multiple sources
- Scraping Abuse: Prevents automated data harvesting by limiting request frequency
Let’s look at a basic limiting scenario: php // Limit user to 60 requests actions per minute
Route::middleware('throttle:60,1')->group(function () { Route::get('/api/data', function () { return response()->json(['message' => 'Success']); }); });
When implementing rate limiting, you can set different thresholds based on:
- Time windows (per minute, hour, or day)
- User roles (admin, regular user, guest)
- IP addresses or API keys
- Client applications or devices
Key Components of Laravel’s Rate Limiting System
Laravel’s rate limiting system operates through three main components that work together seamlessly to manage API requests:
1. Application Cache
The application cache serves as the backbone of Laravel’s rate limiting system. Here’s how it works:
- Stores request counts for each user or IP address
- Maintains timestamps of requests
- Uses Redis or other cache drivers for data persistence
- Automatically cleans up expired entries
2. ThrottleRequests Middleware
API Throttling means to limit the rate of requests or actions to an API to prevent overuse or overload. A throttle controller in Laravel is used to limit the rate of requests to prevent abuse or excessive load on the server.
Laravel has built-in support for limiting requests using the ThrottleRequests middleware, making it simple to set up rate limiting in your app.The ThrottleRequests middleware acts as the gatekeeper for your API endpoints:
php
protected $middlewareGroups = [ 'api' => [ \Laravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful::class, 'throttle:api', \Illuminate\Routing\Middleware\SubstituteBindings::class, ], ];
This middleware:
- Intercepts incoming HTTP requests
- Checks current request counts against defined limits
- Blocks excessive requests when limits are reached
- Returns appropriate HTTP status codes
3. RateLimiter Facade
The RateLimiter facade provides programmatic control over rate limiting:
php
use Illuminate\Support\Facades\RateLimiter; RateLimiter::attempt( 'send-message:' . $user->id, $perMinute = 5, function () { // Send message... } );
The middleware functions in the request lifecycle through these steps:
- Request arrives at your application
- Middleware intercepts the request
- RateLimiter checks cache for existing request counts
- Request either proceeds or gets rejected based on limits
- Cache updates with new request count
Basic Configuration of Rate Limits in Laravel Routes
For developers managing high-traffic applications, implementing PHP rate limiting can prevent servers from being overwhelmed and ensure smooth user experiences
Setting them up in Laravel routes is easy using the RouteServiceProvider. Here’s how to quickly control request limits for your API endpoints:
php
use Illuminate\Cache\RateLimiting\Limit; use Illuminate\Support\Facades\RateLimiter; public function boot() { RateLimiter::for('api', function (Request $request) { return $request->user() ? Limit::perMinute(60)->by($request->user()->id) : Limit::perMinute(30)->by($request->ip()); }); }
This configuration creates different limits for:
- Authenticated users: 60 requests per minute
- Guest users: 30 requests per minute
You can apply these limits to specific routes or groups:
php
Route::middleware('throttle:api')->group(function () { Route::get('/posts', [PostController::class, 'index']); Route::post('/comments', [CommentController::class, 'store']); });
For more granular control, define specific limits per route:
php
// Limit to 5 requests per minute Route::get('/sensitive-data', function () { // Handle the request... })->middleware('throttle:5,1');
// Custom named limiters Route::post('/uploads', function () { // Handle the upload... })->middleware('throttle:uploads');
These rate limits help protect your API while ensuring fair resource distribution among users. The limits are automatically applied, and Laravel takes care of tracking and sending responses when the limit is exceeded.
Advanced Techniques for API Rate Limiting in Laravel
Laravel’s limit and offset controller shines when you need sophisticated control over API access. Let’s dive into advanced implementations that go beyond basic configurations.
Dynamic Rate Limiting Based on User Roles
php
RateLimiter::for('api', function (Request $request) { $user = $request->user(); return match ($user->role) { 'premium' => Limit::perMinute(100), 'business' => Limit::perMinute(50), default => Limit::perMinute(20), }; });
Custom Rate Limiters with Complex Logic
Create powerful rate limiters that consider multiple factors:
php
RateLimiter::for('complex-api', function (Request $request) { return new Limit( key: 'api:' . $request->user()->id, maxAttempts: calculateUserLimit($request), decayMinutes: 1 ); }); function calculateUserLimit($request) { $baseLimit = 30; $subscriptionMultiplier = $request->user()->subscription_level * 2; $timeBasedBonus = (date('H') < 18) ? 10 : 5; return $baseLimit * $subscriptionMultiplier + $timeBasedBonus; }
API Key-Based Rate Limiting
Implement rate limiting for external API consumers:
php
RateLimiter::for('api-key', function (Request $request) { $apiKey = $request->header('X-API-Key'); $keyConfig = ApiKey::where('key', $apiKey)->first(); if (!$keyConfig) { return Limit::none(); } return [ Limit::perMinute($keyConfig->rate_per_minute), Limit::perDay($keyConfig->daily_limit), ]; });
Conditional Rate Limiting
Apply different limits based on endpoint sensitivity:
php
RateLimiter::for('sensitive-endpoint', function (Request $request) { return [ Limit::perMinute(10), // Strict limit for sensitive operations Limit::perHour(100), // Relaxed limit for general operations ]; });
These advanced methods let you adjust API access according to your needs, helping maintain a balance between performance and security.
Handling Responses and Error Codes with Laravel’s Rate Limiting System
When users’ requests exceed their allocated request limits, Laravel automatically triggers a 429 Too Many Requests HTTP status code. This response includes essential headers that help clients understand and manage their rate-limited status.
Here’s what happens behind the scenes:
- The application sends a Retry-After header
- Rate limit information appears in response headers
- Custom error messages can be configured
Let’s look at a typical rate limit exceeded response:
php
return response()->json([ 'message' => 'Too many attempts, please slow down.', 'retry_after' => $seconds, ], 429) ->header('Retry-After', $seconds);
The response headers provide valuable information:
- X-RateLimit-Limit: Maximum attempts allowed
- X-RateLimit-Remaining: Remaining attempts
- Retry-After: Seconds until next attempt is allowed
You can customize these responses in your exception handler:
php
public function render($request, Throwable $exception) { if ($exception instanceof ThrottleRequestsException) { return response()->json([ 'error' => 'API rate limit exceeded', 'reset_time' => $exception->getHeaders()['Retry-After'], ], 429); } }
These response codes and headers help client apps to retry smartly and give users clearer feedback when they hit the rate limit.
Best Practices for Implementing API Rate Limiting in Laravel Applications
Implementing rate limiting strategies in laravel include:
1. Strategic Rate Limit Design
- Set different limits for various endpoints based on their resource intensity
- Implement graduated rate limiting – start with lenient limits and adjust based on usage patterns
- Create separate rate limit pools for read and write operations
2. User-Centric Implementation
- Use unique identifiers like user IDs instead of IP addresses when possible
- Implement clear error messages that guide users on waiting times
- Consider implementing a warning system when users approach their limits
3. Monitoring and Testing Essentials
- Set up automated monitoring for rate limit breaches
- Track rate limit metrics in your application logs
- Create dedicated test scenarios for rate limiting behavior
4. Performance Optimization
php
RateLimiter::for('api', function (Request $request) { return Limit::perMinute(60) ->by($request->user()?->id ?: $request->ip()); });
5. Real-time Adjustments
- Monitor your application’s performance metrics
- Set up alerts for unusual spikes in API usage
- Use Laravel’s built-in events to track rate limit hits
6. Documentation Requirements
- Include rate limit details in API documentation
- Provide clear instructions for handling rate limit errors
- Document any special considerations for premium users or specific endpoints
Remember to regularly review and adjust these configurations based on your application’s growth and user behavior patterns. Consider implementing a dashboard to visualize rate limiting metrics and identify potential bottlenecks or abuse patterns.
Security Benefits of Implementing API Rate Limiting in Laravel Applications
Rate limiting step is an important security measure to protect applications from abuse. Here’s how Laravel’s rate limiting capabilities strengthen your application’s security:
1. Brute Force Prevention
- Blocks repeated login attempts from malicious users
- Limits password guessing attacks on authentication endpoints
- Prevents automated script attacks on sensitive routes
2. Resource Protection
- Guards against scraping attempts by controlling large number of data retrieval requests
- Prevents server overload from excessive API calls
- Maintains stable performance during high-traffic periods
3. Account Security
- Restricts repeated password reset attempts
- Limits account creation requests to prevent spam accounts
- Controls verification email sending frequency
Laravel’s rate limiting system automatically tracks these security threats through its cache system. When suspicious patterns emerge, the system blocks further requests and logs these activities for security analysis. The built-in response headers provide transparency, helping legitimate users understand their request limits while deterring potential attackers.
Conclusion
Mastering limiting is an important defense mechanism for your Laravel applications. By implementing the strategies discussed in this guide, you’re taking a significant step toward building robust, secure, and efficient APIs.
Let’s put these concepts into action by setting up basic rate limits for your routes, creating custom limiters for specific use cases, monitoring your application’s traffic patterns, and adjusting limits based on user roles and needs.
Ready to dive deeper into Laravel development? We’ve got you covered! Visit our Laravel Development Hub for expert insights, tutorials, and best practices.
Remember: A well-implemented rate limiting means not only safeguarding the application but also ensuring fair resource distribution among users. Start implementing these strategies today and watch your API’s reliability and security transform.
Frequently Asked Questions (FAQs)
Yes, Laravel allows you to set unique rate limits for specific routes or groups of endpoints using the throttle middleware with custom parameters.
Laravel’s rate limiter will default to allowing requests if the cache system becomes unavailable, prioritizing application availability over strict rate limiting.
Yes, using a distributed cache system like Redis ensures consistent rate limiting across multiple application servers.
Use Laravel’s ability to define separate rate limiters based on authentication status: php RateLimiter::for(‘api’, function (Request $request) { return $request->user() ? Limit::perMinute(100) : Limit::perMinute(20); });
Yes, you can clear rate limits using the RateLimiter facade’s clear() method with the specific key you want to reset.