Guide on Building eCommerce Platforms with Laravel
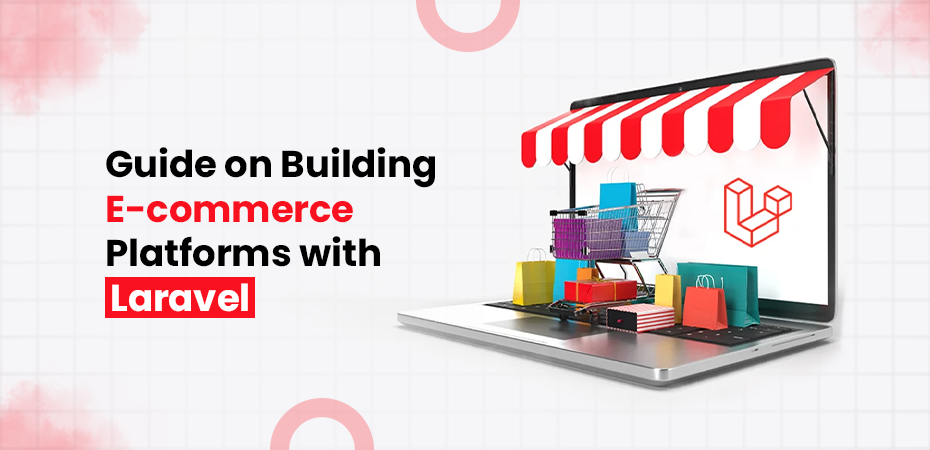
Building a successful eCommerce platform requires a robust and flexible framework, and Laravel has emerged as one of the best choices for developers. In this guide, we will walk you through the process of building an eCommerce platform with Laravel, providing an example of a Laravel project to help you understand each step.
Why Choose Laravel for E-Commerce?
Scalability and Flexibility
Laravel is designed to handle everything from small shops to large-scale eCommerce platforms. It offers a highly flexible structure, making it easy to customize and scale as your business grows. If you’re looking for a laravel sample code of projects or free laravel projects to make your own, you’ll find plenty of resources to get started.
Security
Security is a top priority for any eCommerce site. Laravel comes with built-in security features like password hashing, protection against SQL injection, and CSRF (Cross-Site Request Forgery) protection, ensuring your Laravel website is secure from common vulnerabilities. By following a Laravel tutorial, you can easily implement these features.
Community and Support
With a large and active community, Laravel offers continuous support and frequent updates. Whether you’re facing a technical issue or need help with customization, the Laravel community is always there to help. Exploring laravel tutorials and this blog in laravel can provide you with insights and solutions.
Integration Capabilities
Laravel makes it easy to integrate with various third-party tools and services, from payment gateways to analytics platforms, ensuring your eCommerce site can leverage the best tools available. You can find numerous examples, including laravel ecommerce project github repositories, to guide your integrations.
Setting Up Your Laravel E-Commerce Platform
Prerequisites
Before starting, ensure your system meets the following requirements:
- OS: Ubuntu 16.04 LTS or Higher / Windows 7 or Higher (WAMP / XAMPP)
- Server: Apache 2 or NGINX
- RAM: 4 GB or higher
- PHP: 7.4 or higher
- Processor: 1GHz or higher
- Database: MariaDB 10.2.7 or MySQL 5.7.23 or higher
- Node: 8.11.3 LTS or higher
- Composer: 1.6.5 or higher
Choosing the Right E-Commerce Package
Laravel offers several powerful eCommerce packages. Here are a few popular ones:
- Bagisto: A comprehensive Laravel package that offers multi-featured eCommerce capabilities.
- Aimeos: A full-fledged Laravel eCommerce package suitable for large-scale online stores.
- Vanilo: A modular eCommerce framework for Laravel, allowing high customization.
Select a package that best fits your needs based on your business size and specific requirements. If you’re looking for laravel ecommerce development these packages provide a solid foundation.
Step-by-Step Guide: Building an E-Commerce Platform with Laravel
Building an eCommerce platform with Laravel involves several key steps, each of which contributes to creating a robust and feature-rich online store. Let’s dive into each step in detail.
Installing Laravel
To get started, you’ll need to install Laravel on your development environment. Laravel can be easily installed using Composer, a dependency manager for PHP. Here’s how:
- Install Composer: If you don’t already have Composer installed, download and install it from getcomposer.org.
- Create a New Laravel Project: Run the following command in your terminal: composer create-project laravel/laravel ecommerce-platform
This command will set up a new Laravel project in a directory named ecommerce-platform.
Configuring the Environment
After setting up your Laravel project, the next step is configuring the environment. This involves setting up the .env file, which stores environment-specific variables like database credentials, application URL, and more.
- Set Up Database Connection: Open the .env file and update the following lines with your database details:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=ecommerce
DB_USERNAME=root
DB_PASSWORD=your_password
- Other Configurations: Configure other environment variables like APP_URL, CACHE_DRIVER, and SESSION_DRIVER as per your development and production needs.
Installing an E-Commerce Package (e.g., Bagisto)
Instead of building everything from scratch, you can leverage existing eCommerce packages built on Laravel. Bagisto is one such package that provides a comprehensive set of features right out of the box.
- Install Bagisto: Navigate to your Laravel project’s root directory and run: composer create-project bagisto/bagisto
- Configure Bagisto: Once installed, update the .env file with necessary configurations like database credentials. Bagisto also requires you to set up the email credentials for sending transactional emails.
Database Migrations and Seeding
Migrations in Laravel allow you to define your database schema and apply changes over time. Seeding, on the other hand, populates your database with initial data.
- Run Migrations: Execute the following command to create the necessary database tables: php artisan migrate
- Seed the Database: Seed the database with initial data using: php artisan db:seed
This will populate your database with default values necessary for the eCommerce platform to function.
Setting Up the Admin Panel
A robust admin panel is essential for managing your eCommerce platform. Bagisto comes with a built-in admin panel that allows you to manage products, orders, customers, and more.
- Access the Admin Panel: Once Bagisto is installed, access the admin panel by navigating to: http://yourdomain.com/admin/login
- Customizing the Admin Panel: You can customize the admin panel by modifying the views located in the resources/views/admin directory. Laravel’s Blade templating engine makes it easy to customize these views according to your needs.
Building and Customizing the Front-End
The front-end of your eCommerce platform is the face of your business. Laravel integrates seamlessly with modern front-end frameworks like Vue.js, React, and Angular. Integrating laravel/ui can significantly speed up the development process, especially when building the ecommerce website (frontend).
- Using Blade Templating: Laravel’s Blade templating engine is powerful and easy to use. Create and customize views in the resources/views directory to design your storefront.
- Integrating with Vue.js: For a more dynamic user experience, consider integrating Vue.js. Laravel provides built-in support for Vue.js, making it easy to create reactive components and single-page applications (SPAs).
Implementing Product Management
Product management is at the core of any eCommerce platform. Bagisto and other Laravel packages typically come with built-in support for product catalogs, but you can extend these functionalities to suit your needs.
- Product Categories: Set up product categories to organize your inventory.
- Product Attributes: Define attributes like size, color, and material to offer product variants.
- Inventory Management: Implement inventory management to keep track of stock levels and automate stock updates.
Advanced Features and Customizations
Once the basic eCommerce platform is up and running, it’s time to explore advanced features and customizations that can enhance the user experience and provide additional functionality.
Adding Multi-Vendor Support
Multi-vendor functionality allows multiple sellers to register and sell their products on your platform. This can transform your eCommerce site into a marketplace, similar to Amazon or eBay.
- Vendor Registration: Implement a system where vendors can sign up, create profiles, and list their products.
- Vendor Dashboard: Provide vendors with a dashboard to manage their products, orders, and sales.
- Commission Structures: Set up commission structures where the platform owner earns a percentage of each sale.
Inventory Management
Managing inventory is crucial, especially for platforms handling multiple warehouses or large inventories.
- Multi-Warehouse Management: Bagisto and other Laravel eCommerce packages often support multi-warehouse management, allowing you to manage stock across different locations.
- Automated Stock Updates: Implement automated stock updates that reflect real-time inventory levels across your warehouses.
- Stock Notifications: Set up notifications for low stock levels to prevent stockouts.
Integrating Payment Gateways
Accepting payments securely is a critical aspect of any eCommerce platform. Laravel makes it easy to integrate various payment gateways.
- Stripe Integration: Stripe is a popular choice for handling online payments. Use Laravel Cashier, a package that simplifies Stripe integration, to manage subscriptions, payment intents, and more.
- PayPal Integration: PayPal is another widely used payment gateway. Integrate PayPal using Laravel’s service provider or a third-party package.
- Custom Payment Methods: If your platform requires specific payment methods, Laravel’s flexible architecture allows you to build and integrate custom payment solutions.
Customizing the Checkout Process
The checkout process is where customers complete their purchase, so it’s vital to make it as smooth and user-friendly as possible.
- One-Page Checkout: Implement a one-page checkout process to reduce friction and improve conversion rates.
- Guest Checkout: Allow customers to checkout as guests without requiring an account, which can help reduce cart abandonment.
- Custom Shipping Methods: Offer various shipping options, including standard, express, and same-day delivery, based on customer preferences.
SEO Optimization
Search engine optimization (SEO) is essential for driving organic traffic to your eCommerce platform. Laravel offers several tools and packages to help with SEO.
- Clean URLs: Ensure your URLs are clean and descriptive, making them more search-engine friendly.
- Meta Tags: Use Laravel’s Blade templates to dynamically generate meta tags for each product and category page.
- Sitemaps: Generate XML sitemaps to help search engines crawl and index your site more efficiently.
Deploying Your Laravel E-Commerce Platform
Once development and testing are complete, it’s time to deploy your eCommerce platform. Laravel Forge or other deployment tools can help automate the deployment process, making it easier to push your ‘Laravel site’ live.
1. Preparing the Server
Ensure your server is configured with all necessary software, including PHP, a web server (Apache or Nginx), and a database (MySQL or MariaDB).
2. Setting Up CI/CD Pipeline
A CI/CD (Continuous Integration/Continuous Deployment) pipeline automates the process of testing and deploying your code. Tools like Jenkins, GitLab CI, or GitHub Actions can be used to set up your CI/CD pipeline.
3. Monitoring and Maintenance
After deployment, regular monitoring and maintenance are crucial to keep your eCommerce platform running smoothly.
- Performance Monitoring: Use tools like New Relic or Laravel Telescope to monitor your platform’s performance.
- Security Updates: Regularly apply security patches and updates to ensure your platform remains secure.
- Backup Strategies: Implement regular backups to protect against data loss in case of server failure or other issues.
Conclusion
Building an eCommerce PHP platform with Laravel is a rewarding experience that provides you with a scalable, secure, and customizable solution. Laravel has become the go-to framework for developers building PHP eCommerce platforms, offering a robust and scalable solution for businesses of all sizes.
By following the steps outlined in this guide and exploring sites built with Laravel for inspiration, you can create a powerful eCommerce platform tailored to your business needs. Whether you’re a beginner or an experienced developer, Laravel offers all the tools and resources you need to succeed in the world of eCommerce.