Building Cloud Agnostic Applications with Laravel
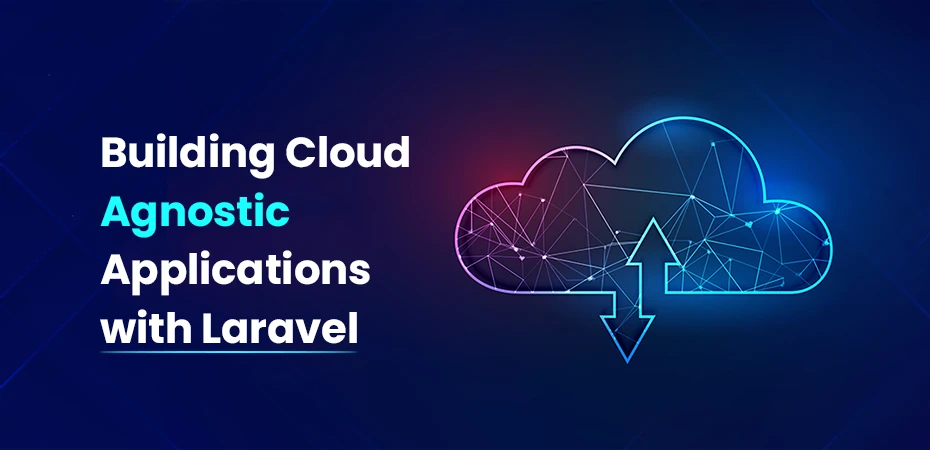
Cloud and platform agnostic applications are a big change in software development. Platform agnostic means that a software application or system is designed to work on any platform, such as different operating systems or cloud providers, without being tied to a specific one.
Cloud agnostic means that an application or system is designed to run on any cloud platform without being tied to a specific provider, such as AWS, Google Cloud, or Azure. It allows flexibility to switch between different cloud services without major changes to the application.
Laravel is a great tool for building these flexible apps. It’s a strong PHP framework that gives you the freedom to create cloud-agnostic solutions. Laravel’s clean code, many features, and strong community make it a great choice for developers.
Cloud agnostic approach has many benefits. It offers more flexibility, cost effectiveness, and better disaster recovery. But there are challenges as well. Developers need to make sure performance stays good on different platforms, meet security rules, and deal with each cloud provider’s unique features.
Understanding Cloud Agnostic Applications
An agnostic platform is a type of software that can work on different systems without being limited to just one. Cloud-agnostic apps are different from cloud-native apps because cloud-native apps are made for one specific cloud service, while cloud-agnostic apps can run on many different cloud services.
Think of cloud or platform agnostic apps as universal travelers—they can easily adapt to any environment. This flexibility comes from separating the app from specific cloud services, making it able to run anywhere.
Cloud Agnostic vs. Cloud Native: Key Differences
- Cloud Agnostic: Uses standard technologies and protocols.
- Cloud Native: Relies on specific cloud provider features.
Benefits of Avoiding Vendor Lock-in:
- Less dependency on one provider’s pricing.
- Ability to choose the best services from different platforms.
- More negotiating power with cloud providers.
- Easier to move workloads when business needs change.
Benefits and Challenges in Developing Cloud Agnostic Applications with Laravel
Building agnostic software and applications with Laravel offers several benefits and challenges that impact the development process. Let’s explore these through real-world examples and practical scenarios.
1. Enhanced Flexibility and Portability
Laravel’s strong design makes it easy to move applications between different cloud services. For example, Netflix uses both AWS and Google Cloud, switching between them depending on performance and cost.
Laravel also works well with hybrid cloud setups. Spotify uses both Google Cloud and AWS to get the best features from each, while keeping things running smoothly. This flexibility helps companies improve their cloud strategy.
Key Benefits of Using Laravel:
- Resource Optimization: Laravel’s service container lets you easily switch cloud services without changing the code.
- Database Flexibility: Laravel’s database layer makes it simple to switch between different database providers.
- Storage Adaptability: Built-in filesystem support works with various cloud storage options.
Business Advantages of Multi-Cloud Strategies:
- 99.99% uptime across all regions.
- 30% reduction in operational costs.
- Better disaster recovery.
During busy times, Laravel’s flexibility lets businesses spread work across different cloud services to improve performance. Its features help scale up smoothly to handle high demand. Airbnb is an example of a company that successfully uses Laravel with a multi-cloud approach.
2. Cost Reduction Strategies
Building applications that are agnostic to the cloud with Laravel can help save money. By using different cloud providers for different needs, businesses can cut costs.
For example, your Laravel app might use AWS for cheap computing and Google Cloud for cheaper storage. This way, you get the best prices from each provider.
Here are some tips for saving costs:
- Use spot instances for non-essential tasks.
- Set up auto-scaling based on real usage.
- Use serverless functions for tasks that only happen sometimes.
Laravel makes it easy to apply these strategies. Its queue system can send tasks to different cloud providers, saving money based on their prices.
Cost-saving features include:
- Laravel’s cache drivers work with various cloud storage.
- The queue system supports different cloud-based message services.
- Database connections can be balanced across providers for better pricing.
Organizations using these methods often save 20-30% compared to using just one cloud. As your app grows, these savings increase, making cloud-agnostic architecture a smart choice for the future.
3. Improved Disaster Recovery Capabilities
Building cloud-agnostic applications with Laravel helps companies create better disaster recovery plans by using multiple cloud services. This flexibility makes sure your data is safe, as the application can quickly switch to another provider if needed.
Practical Example: Backing Up Databases on Multiple Clouds
You can store primary database backups on AWS S3 and secondary backups on Google Cloud Storage. Laravel’s built-in filesystem abstraction makes it simple:
Storage::disk(‘s3’)->put(‘backup.sql’, $backup);
Storage::disk(‘gcs’)->put(‘backup.sql’, $backup);
This approach ensures your backups are secure and accessible across different cloud environments.
Security Benefits of a Multi-Cloud Approach
Using multiple cloud providers boosts security through diversity. Each provider brings its own strengths:
- AWS: Strong encryption and identity management.
- Google Cloud: Advanced threat detection.
- Azure: Extensive compliance certifications.
You can combine Laravel’s security features with these provider-specific tools for enhanced protection. For example, you can implement rate limiting across different cloud environments:
RateLimiter::for('backups', function (Request $request) { return Limit::perMinute(1)->by($request->user()->id); });
This multi-layered security strategy creates a more resilient system, capable of handling various threats and failures.
4. Managing Complexity Across Clouds
Managing Multiple Cloud Environments: Challenges for Laravel Developers
Working with multiple cloud environments can be tricky. It adds complexity to deployment, monitoring, and maintenance.
Configuration Management
A big challenge is managing settings across different cloud services with different setups. One solution is to create layers in Laravel that let the app work with each provider without needing to change the code a lot.
Simplifying Multi-Cloud Deployments
Docker and Kubernetes are key to simplifying multi-cloud setups. These tools help ensure consistency and scalability across cloud environments for Laravel applications:
- Docker: Packages applications with all dependencies, making them run the same way on any cloud platform.
- Kubernetes: Manages and scales containerized applications across multiple clouds.
- Infrastructure-as-Code: Automates deployment, making processes more efficient.
- Service Mesh: Manages communication between services, improving coordination.
Monitoring Application Performance
To monitor performance across different cloud services, it’s important to have strong tracking tools. Tools like Prometheus and Grafana work well with Laravel, giving real-time information about system health and performance.
Version Control Strategies
Managing configurations across multiple clouds requires effective version control. Using Laravel’s environment configuration system alongside Git branches for each cloud helps keep deployment settings organized and controlled. This ensures consistency and reduces errors during deployment.
5. Ensuring Security Compliance with Multiple Clouds
Security Compliance Across Multiple Clouds
Managing security can be tough when using multiple cloud services because each one has its own security rules and methods. But, there are important practices that can help keep your Laravel app secure across different clouds.
Unified Access Control
A strong security system starts with having one clear way to manage access. You can do this by combining Laravel’s built-in login system with the identity tools from each cloud service. This creates a consistent security layer across all clouds, making it easier to manage who can access the app.
Regular Security Audits
Regular security checks are important to stay compliant. Automated tools can help monitor cloud systems for problems, track how data moves, make sure encryption is used, and confirm that data protection laws are followed.
The laravel-audit package tracks changes in your app, no matter which cloud you use, and can work with other cloud logging tools to create a full audit trail.
Data Encryption
Encrypting data is crucial. Use Laravel’s built-in encryption alongside your cloud provider’s encryption services. This double-layer approach keeps your data safe, even when moving between clouds.
Centralized Security Monitoring
Consider using a Security Information and Event Management (SIEM) system. This system centralizes security monitoring across all cloud platforms, making it easier to spot and respond to threats in real-time.
Building Cloud Agnostic Applications with Laravel: A Step-by-Step Guide
Setting Up Your Development Environment
To start building cloud-agnostic applications with Laravel, you need the right tools:
- PHP 8.0 or higher: Ensure your PHP version is up-to-date.
- Composer: Use it for managing dependencies.
- Docker: Containerize your application for portability across clouds.
- Git: Use Git for version control to track changes and manage deployments.
These tools lay the foundation for a smooth development process and make your Laravel app adaptable to different cloud environments.
Creating a Clean Architecture
To make your application cloud-agnostic, start with a clean architecture. Laravel’s service container and dependency injection help you create a decoupled structure that can easily switch between cloud providers.
Implementing Abstract Services
Create service classes that work with different cloud providers. For example, a CloudStorageService class can use an abstract interface to handle interactions with various cloud platforms:
namespace App\Services; class CloudStorageService implements StorageServiceInterface { private $provider; public function __construct(CloudProviderInterface $provider) { $this->provider = $provider; } }
This approach allows you to easily swap out cloud services without changing your core application logic, making your app more portable and flexible.
Using Environment Variables
Laravel’s .env file makes it easy to manage different configurations for various cloud environments. You can define settings like storage, cache, and queue drivers, which will change based on the cloud provider you’re using.
This allows you to easily switch configurations by changing values in the .env file, making your application adaptable to different cloud platforms without modifying the core code.
Database Abstraction
Laravel’s database abstraction layer allows easy switching between different database providers. You can use the same code to interact with multiple databases by simply changing the connection:
use Illuminate\Support\Facades\DB; DB::connection('mysql') ->table('users') ->get(); DB::connection('postgres') ->table('users') ->get();
This flexibility ensures your application can adapt to different database systems without major changes to your queries.
Service Providers
In Laravel, you can register cloud-agnostic services in the service container, making it easy to switch between cloud providers. For example, a CloudServiceProvider can bind storage services based on the provider set in the configuration:
namespace App\Providers; use Illuminate\Support\ServiceProvider; class CloudServiceProvider extends ServiceProvider { public function register() { $this->app->bind(StorageServiceInterface::class, function ($app) { return new CloudStorageService( $app->make(config('services.cloud.provider')) ); }); } }
This way, you can easily swap cloud services by changing the configuration, without altering the core logic of your application.
Leveraging Open Source Tools with Laravel
Laravel’s Adaptability with Containerization
Laravel works seamlessly with tools like Docker, which helps you build apps that can run on any cloud. Docker lets you put your Laravel app and everything it needs into small packages called containers, so it works the same on different cloud platforms.
Kubernetes for Container Orchestration
Kubernetes enhances Docker by managing container deployment, scaling, and operations across clusters. For Laravel apps, Kubernetes offers several advantages:
- Auto-scaling: Adjusts resources based on traffic.
- Load balancing: Distributes requests across multiple containers (pods).
- Self-healing: Automatically replaces failed containers.
- Rolling updates: Deploy new versions with no downtime.
Tools for Easier Container Integration
Laravel Sail is a lightweight command-line interface for Docker, simplifying container management in Laravel projects.
It ensures consistency between development and production. Your Laravel app stays cloud-agnostic. It runs smoothly across different cloud platforms. No major configuration changes are needed.
Containerization, Microservices, and Application Performance Monitoring
Containerization simplifies cloud-agnostic system development by creating consistent environments across platforms. With containers, your Laravel app includes all dependencies, ensuring reliable deployments and eliminating the “it works on my machine” issue.
A microservices architecture breaks your Laravel app into smaller, independent services, each handling specific business functions. These services communicate through APIs, allowing independent scaling and deployment without affecting the whole app.
This makes it easier to make changes, improve performance, and keep the system strong. In a setup with multiple services, tools like New Relic, Datadog, or Laravel Telescope help track how fast the services respond, how much resources they use, how often errors happen, and how users are experiencing the app.
Conclusion
Platform agnosticism refers to the design or approach where software, applications, or systems are not dependent on a specific platform, such as a particular operating system, cloud provider, or hardware. Building cloud-agnostic applications with Laravel opens up endless possibilities for developers and businesses.
Laravel’s powerful ecosystem provides a solid foundation for creating flexible, portable apps that work in any cloud environment. With its extensive features and the right architecture, you can build applications that are independent of specific cloud providers.
Ready to level up your Laravel development? Our team of expert Laravel developers specializes in creating cloud-agnostic apps that scale effortlessly across different platforms. Hire Dedicated Laravel Developers to see how we can help you build resilient, future-proof applications.
Frequently Asked Questions (FAQs)
Yes, you can refactor your existing Laravel application by implementing repository patterns, containerization, and abstracting cloud-specific services.
While abstraction layers might add minimal overhead, proper implementation and optimization can maintain high performance levels across different cloud platforms.
Laravel applications work seamlessly with major providers like AWS, Google Cloud, and Azure. The choice depends on your specific needs and budget.
While not mandatory, containerization with Docker simplifies deployment and ensures consistency across different cloud environments.
Use Laravel’s database abstraction layer, implement robust backup strategies, and consider distributed database solutions for data synchronization.