CRUD Operations in Laravel: The Ultimate Step-by-Step Tutorial
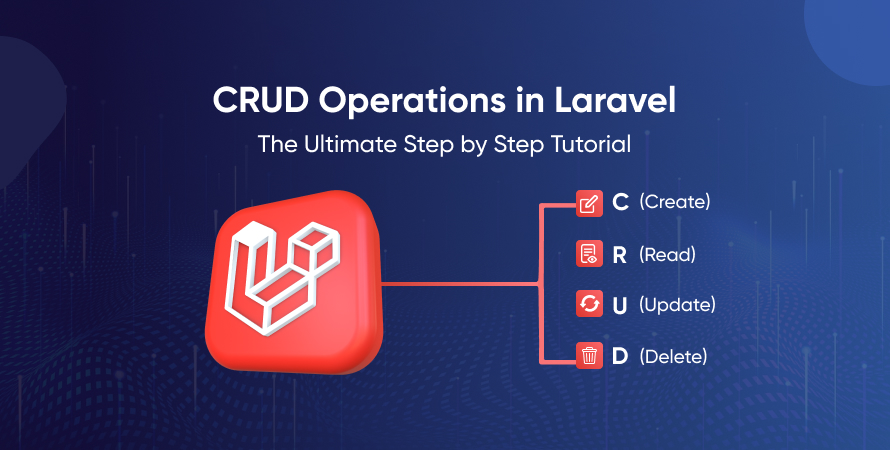
CRUD operations—Create, Read, Update, Delete—are essential for web development. They allow you to effectively manage and manipulate data in your applications.
Laravel is a popular PHP framework used for creating powerful web applications. Its simple syntax and robust features make common tasks easier, which is why many developers love it.
In this tutorial, you’ll learn how to:
- Set up a new Laravel project
- Configure database connections
- Create models and migrations
- Generate resource controllers
- Define application routes
- Design user interfaces with Blade templates
- Validate user input
- Test and debug your application
By mastering CRUD operations in Laravel, you’ll be well-equipped to handle data management efficiently in any web application.
Setting Up Your Development Environment
System Requirements for Laravel Installation
To install Laravel, ensure your system meets the following requirements:
- PHP version 7.3 or higher
- Composer (Dependency Manager for PHP)
- OpenSSL PHP Extension
- PDO PHP Extension
- Mbstring PHP Extension
- Tokenizer PHP Extension
- XML PHP Extension
Step-by-Step Guide to Install Laravel Using Composer
- Install Composer: bash php -r “copy(‘https://getcomposer.org/installer’, ‘composer-setup.php’);” php composer-setup.php php -r “unlink(‘composer-setup.php’);”
- Verify Composer Installation: bash composer –version
- Create a New Laravel Project: bash composer create-project –prefer-dist laravel/laravel my-laravel-app
Setting Up XAMPP for Local Development
Installing XAMPP
Download and install XAMPP suitable for your OS.
Launch XAMPP Control Panel.
Configuring MySQL and Apache for Laravel Projects
Start Apache and MySQL from the XAMPP Control Panel.
Create a new database via phpMyAdmin:
Open http://localhost/phpmyadmin
Click on “New” and create a database, e.g., laravel_db.
Configure .env File in Laravel Project: plaintext DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_db DB_USERNAME=root DB_PASSWORD=
This setup allows you to utilize Laravel’s powerful features efficiently on your local machine, ensuring you have everything ready for creating robust web applications.
In case you’re considering hiring professional help, such as a dedicated Laravel developer, remember to review their privacy policy to ensure compliance and build trust.
Creating Your First Laravel Project
Command to Create a New Laravel Project
To create a new Laravel application, open your terminal and navigate to the directory where you want to set up your project. Run the following command:
bash composer create-project –prefer-dist laravel/laravel myLaravelApp
Replace myLaravelApp with your desired project name.
Configuring the .env File for Database Connection
After creating your Laravel project, configure the database connection settings in the .env file located in the root directory of your project. Open this file and update the following lines to match your database credentials:
env DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=your_database_name DB_USERNAME=your_username DB_PASSWORD=your_password
Replace your_database_name, your_username, and your_password with your MySQL database details.
Creating a New Database in MySQL
Before connecting Laravel to your database, you need to create a new database in MySQL. Follow these steps:
Open phpMyAdmin or any MySQL client.
Click on “New” to create a new database.
Enter a name for your database that matches the DB_DATABASE value in the .env file.
Click “Create”.
By following these steps, you have successfully set up a new Laravel project and configured it to connect to your MySQL database.
If you encounter any issues during this process or need further assistance with your Laravel application, consider reaching out for professional help. You can contact us for Laravel developer services and support. Our expert team is ready to assist you with any questions or project needs you may have.
Working with Eloquent ORM: The Power of Database Interactions in Laravel
What is Eloquent ORM?
Eloquent ORM (Object-Relational Mapping) is Laravel’s built-in library for database interactions. It enables you to work with databases using an object-oriented approach, simplifying the process of performing CRUD operations in Laravel.
Benefits of Using Eloquent for Database Interactions
Here are some benefits of using Eloquent for database interactions:
- Elegant Syntax: Eloquent provides an intuitive and expressive syntax that makes database queries easy to read and write.
- Active Record Implementation: Each model corresponds to a single database table, allowing you to interact with data directly through model instances.
- Relationships Management: Easily define and manage relationships like one-to-many, many-to-many, and polymorphic relationships between tables.
- Data Validation: Built-in methods help validate and sanitize data before performing operations, enhancing data integrity.
Basic Eloquent Querying Methods
Some fundamental methods that you will frequently use include:
- Retrieving All Records: php $users = User::all();
- Finding a Record by Primary Key: php $user = User::find($id);
- Inserting New Records: php $user = new User; $user->name = ‘John Doe’; $user->email = ‘john@example.com’; $user->save();
- Updating Existing Records: php $user = User::find($id); $user->name = ‘Jane Doe’; $user->save();
- Deleting Records: php $user = User::find($id); $user->delete();
Eloquent makes it easy to handle these basic CRUD operations while maintaining clean and manageable code. You get the advantage of working with a robust ORM system that enhances productivity and ensures consistency across your application.
Building Models and Migrations: Structuring Your Database Tables with Ease
Models play a crucial role in Laravel’s MVC (Model-View-Controller) architecture. They act as an intermediary between the application’s database and its logic, enabling you to interact with your data effortlessly.
Creating Models and Migrations
To create models and migrations, Artisan commands come in handy. Use the following command to generate both a model and migration simultaneously:
bash php artisan make:model ModelName -m
Replace ModelName with the desired name of your model. This command creates a new model file in the app/Models directory and a corresponding migration file in the database/migrations directory.
Defining Table Structures in Migrations
Defining table structures in migrations is straightforward. Open the generated migration file and use Laravel’s Schema Builder to define your table schema. Here’s an example:
php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema;
class CreateModelNameTable extends Migration { public function up() { Schema::create(‘model_name’, function (Blueprint $table) { $table->id(); $table->string(‘column_name’); $table->timestamps(); }); }
public function down()
{
Schema::dropIfExists(‘model_name’);
}
}
In this example, replace model_name with your table name and column_name with your desired column names. The up() method defines the schema for creating the table, while the down() method provides instructions for rolling back the migration.
Using these tools, you can efficiently structure your database tables, ensuring that your application’s data layer is both robust and flexible.
Crafting Resource Controllers: Simplifying CRUD Logic with Convenience Methods
Resource controllers in Laravel streamline CRUD operations by providing a standardized way to handle common tasks. They bundle methods for creating, reading, updating, and deleting data into a single, manageable class.
Creating a Resource Controller
To generate controllers like PostController or NoteController, you use the Artisan command:
bash php artisan make:controller PostController –resource php artisan make:controller NoteController –resource
This command sets up a resource controller with predefined methods corresponding to each CRUD operation.
Overview of Key Methods
- index(): Fetches all records from the database.
- create(): Displays a form for creating a new record.
- store(Request $request): Validates input data and saves the new record to the database.
- show($id): Retrieves and displays a specific record based on its ID.
- edit($id): Shows a form pre-filled with data of an existing record for editing.
- update(Request $request, $id): Validates updated data and saves changes to the specified record.
- destroy($id): Deletes a specific record from the database.
Resource controllers simplify your code, making it more readable and maintainable. Each method is mapped to a corresponding HTTP request type (GET, POST, PUT, DELETE), allowing seamless data management.
Defining Routes for Seamless Data Flow: Connecting URLs to Controller Actions Seamlessly
Setting up routes in Laravel is crucial for linking your application’s URLs to the appropriate controller actions. To define routes, navigate to the routes/web.php file and use the Route facade.
Setting Up Routes in web.php
In web.php, you can define routes using various methods such as get, post, put, and delete. For example:
php Route::get(‘/posts’, [PostController::class, ‘index’]); Route::post(‘/posts’, [PostController::class, ‘store’]); Route::get(‘/posts/{id}’, [PostController::class, ‘show’]); Route::put(‘/posts/{id}’, [PostController::class, ‘update’]); Route::delete(‘/posts/{id}’, [PostController::class, ‘destroy’]);
Understanding Route Parameters and Naming Conventions
Routes often require parameters to identify specific resources. In the above example, {id} is a route parameter. You can access this parameter within your controller methods. Naming conventions are important for readability and maintainability. Use meaningful names for your routes to make them easier to understand.
Using Resource Routing for Cleaner Code
Laravel simplifies routing with resource routing. Instead of defining each route individually, you can use the following single line in web.php:
php Route::resource(‘posts’, PostController::class);
This command automatically generates all CRUD-related routes, mapping them to the corresponding controller methods (index, create, store, etc.). This approach keeps your code cleaner and more manageable.
Understanding and defining routes effectively ensures that your Laravel application operates smoothly by connecting URLs seamlessly to their respective controller actions. If you encounter any issues or need further assistance with your Laravel project, feel free to reach out through our contact page. We’re here to help!
Creating Views with Blade Templates: Designing User Interfaces Efficiently
Blade templates are Laravel’s powerful and flexible templating engine. They allow you to create dynamic and reusable views that make your application easy to manage and scale.
Introduction to Blade Templating Engine
Blade templates are stored in the resources/views directory and use the .blade.php file extension. Laravel automatically compiles these files into plain PHP when they are rendered, ensuring optimal performance while maintaining readability.
Structuring Blade Files for CRUD Functionalities
To structure your Blade files effectively, consider creating a dedicated folder for each resource. For example, if you have a Post model, you might create a posts directory within resources/views. Inside this folder, you’ll generate separate view files for each CRUD operation:
- index.blade.php: Lists all records.
- create.blade.php: Shows a form to create a new record.
- edit.blade.php: Displays a form pre-filled with an existing record’s data.
- show.blade.php: Displays a single record’s details.
Creating Forms for Data Input (Create/Edit) and Displaying Records (Index/Show)
Here’s how to create forms and display records using Blade:
Creating a Form for Data Input
To create a form for adding or editing posts:
php
<label for=”content”>Content</label>
<textarea id=”content” name=”content” required></textarea>
<button type=”submit”>Submit</button>
The @csrf directive generates a CSRF token field, which is necessary for form security.
Displaying Records (Index/Show)
To list all posts:
php
@foreach ($posts as $post) {{ $post->title }} {{ $post->content }} View Edit
<form action=”{{ route(‘posts.destroy’, $post->id) }}” method=”POST”>
@csrf
@method(‘DELETE’)
<button type=”submit”>Delete</button>
</form>
</div>
@endforeach
Using Blade templates, you can efficiently manage your application’s views while maintaining clean and organized code.
Validating User Input: Ensuring Data Integrity with Form Request Validation
Validation is crucial when handling user input to maintain data integrity and security in your application. Laravel’s Form Request Validation offers a clean and efficient way to manage validation logic.
Importance of Validation
- Data Integrity: Prevents invalid or malicious data from being stored in the database.
- User Experience: Provides immediate feedback to users when they submit incorrect information.
- Security: Protects against common vulnerabilities like SQL injection and XSS attacks.
Using Form Request Classes
Form Request classes encapsulate validation logic, making your controllers cleaner and more readable. To create a Form Request class, use the Artisan command:
bash php artisan make:request StorePostRequest
This generates a new request class in the app/Http/Requests directory. Inside this class, you can define validation rules and custom messages:
php public function rules() { return [ ‘title’ => ‘required|max:255’, ‘content’ => ‘required’, ]; }
public function messages() { return [ ‘title.required’ => ‘A title is required’, ‘content.required’ => ‘Content is required’, ]; }
In your controller, instead of manually validating input, inject the request class:
php public function store(StorePostRequest $request) { // The validated data is automatically available $validated = $request->validated(); Post::create($validated); }
Common Validation Rules
Laravel provides a variety of built-in validation rules that can be used in CRUD forms:
- required: Ensures the field is not empty.
- max:value: Limits the maximum length of a string.
- email: Validates the input as a valid email address.
- unique: table,column: Ensures the value is unique in a specific table column.
- confirmed: Checks if two fields match (useful for password confirmation).
These rules can be combined for robust validation logic, ensuring that your application’s data remains consistent and secure.
By leveraging Laravel’s Form Request Validation, you streamline the process of validating user input, keeping your code organized and maintainable.
Testing Your Application: Running it Locally and Debugging Issues
Running your Laravel application locally is essential to verify that your CRUD operations function as expected. Laravel offers a built-in development server, which you can start using the following Artisan command:
bash php artisan serve
Once the server is running, navigate to http://localhost:8000 in your web browser. Here, you can interact with your application and test the CRUD functionalities:
- Create: Fill out and submit forms to add new records.
- Read: Navigate through lists of records and view individual entries.
- Update: Edit existing records using pre-filled forms.
- Delete: Remove records and ensure they no longer appear in listings.
Testing through the browser allows you to experience the application as an end-user would, ensuring every feature works smoothly.
Debugging Common Issues
While testing, you might encounter several common issues:
- Database Connection Errors: Ensure your .env file is correctly configured with the right database credentials.
- 404 Not Found Errors: Double-check route definitions in web.php and ensure they match your controller methods.
- Validation Failures: Confirm that validation rules in Form Request classes are correctly defined and applied.
Utilize Laravel’s built-in debugging tools like error messages and logging features to identify and resolve issues promptly. The storage/logs/laravel.log file provides detailed error logs which can be invaluable for troubleshooting.
Conclusion
Mastering CRUD operations in Laravel is a crucial step in becoming proficient with this framework. With the knowledge gained from this Laravel CRUD tutorial, you can now:
- Create new records
- Read existing data
- Update records
- Delete entries
These skills lay a strong foundation for developing dynamic web applications.
Encouraged to push your boundaries, delve into more advanced features of Laravel:
- Implement middleware for request filtering.
- Explore queues and jobs for background processing.
- Utilize Laravel’s API resources for building RESTful APIs.
Each new feature you learn will enhance your ability to build more efficient and scalable applications. Continue to experiment and grow, as the Laravel ecosystem offers endless possibilities.