How to Secure Laravel Applications: Best Practices and Tips?
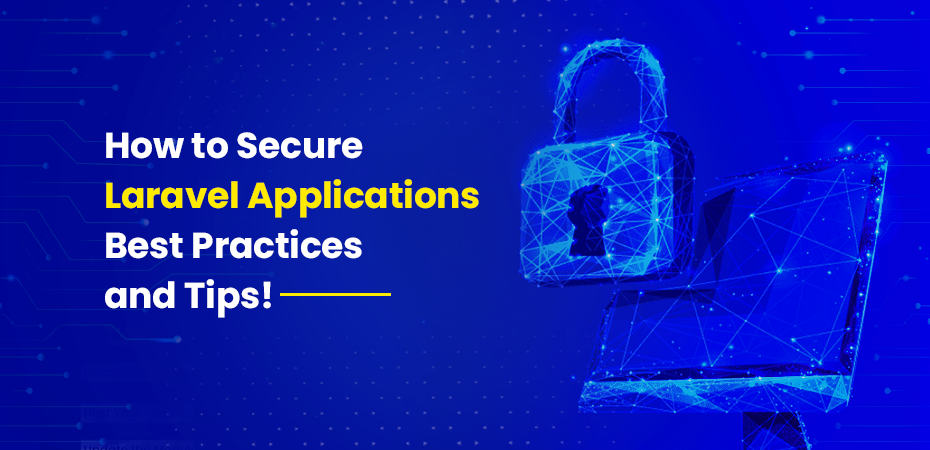
Laravel is a powerful and user-friendly PHP framework that stands out for its simplicity and wide range of features. It has become extremely popular among developers who want to create modern web applications quickly and effectively. With its clear syntax and comprehensive set of tools, Laravel makes tasks like routing, authentication, and caching much easier.
But just like any other web application framework, security is a major concern. It’s crucial to protect Laravel applications from common vulnerabilities such as SQL injection, Cross-Site Request Forgery (CSRF) attacks, and Cross-Site Scripting (XSS) in order to safeguard sensitive information and maintain the trust of users.
This article will explore the Laravel best practices for securing Laravel applications. We will discuss various techniques and strategies that can help you defend against potential threats and build more secure applications.
By the end of this article, you will have a thorough understanding of how to secure your Laravel applications. Whether you’re looking for guidance on creating a useful tool with Laravel, seeking assistance with specific issues, or wanting to protect your application from exploitation scenarios, this guide has everything you need.
Keeping Laravel Updated
Staying current with Laravel updates is crucial for maintaining the security and performance of your application. Regular updates ensure that you benefit from the latest security patches and bug fixes, protecting your application from vulnerabilities that could be exploited by malicious actors.
Importance of Regular Updates
Updating Laravel regularly helps to:
- Patch known security vulnerabilities.
- Fix bugs that could be exploited.
- Improve the overall stability and performance of your application.
Ignoring updates can leave your application susceptible to attacks. For instance, a known vulnerability in an outdated version of Laravel can be easily targeted if not patched.
How to Check for Updates?
To keep Laravel updated, you can follow these steps:
- Composer: Use Composer to check for available Laravel exploit updates. Run: bash composer outdated laravel/framework.
- Official Documentation: Regularly visit the Laravel official documentation and release notes to stay informed about new versions and changes.
- Security Advisories: Subscribe to Laravel’s security advisories on GitHub to receive notifications about any potential security issues.
By staying proactive with updates, you ensure that your Laravel application remains secure, stable, and up-to-date with the latest features.
Strong Authentication Mechanisms
Implementing Complex Passwords and Account Lockout Mechanisms
A robust authentication system begins with strong password policies. Encourage users to create complex passwords by enforcing rules such as a minimum length, the inclusion of special characters, numbers, and uppercase letters. Additionally, implementing account lockout mechanisms is essential to defend against brute-force attacks. By limiting the number of login attempts and temporarily locking accounts after several failed attempts, you can significantly reduce the likelihood of unauthorized access.
php // Example: Laravel validation rule for strong passwords $request->validate([ ‘password’ => [‘required’, ‘string’, ‘min:8’, ‘regex:/[a-z]/’, ‘regex:/[A-Z]/’, ‘regex:/[0-9]/’, ‘regex:/[@$!%*?&]/’], ]);
Using Laravel Jetstream for Two-Factor Authentication (2FA)
Two-Factor Authentication (2FA) enhances security by requiring a second form of verification in addition to a password. Laravel Jetstream provides seamless integration for 2FA using tools like Google Authenticator or SMS-based OTPs. This additional layer makes it exponentially harder for attackers to gain access, even if they obtain user credentials.
php // Example: Enabling 2FA in Laravel Jetstream $user->enableTwoFactorAuthentication();
Exploring Passwordless Authentication Options
Passwordless authentication offers an innovative approach by eliminating traditional passwords altogether. Options include magic links sent via email or biometric logins like fingerprint or facial recognition. These methods not only improve security but also enhance user experience by simplifying the login process.
- Magic Links: Users receive a unique link via email that grants access upon clicking.
- Biometric Login: Utilizes device-specific features like Touch ID or Face ID.
php // Example: Sending magic link using Laravel $url = URL::temporarySignedRoute( ‘login.magic-link’, now()->addMinutes(30), [‘user’ => $user->id] ); Mail::to($user->email)->send(new MagicLinkMail($url));
Combining these strategies ensures a fortified authentication system, safeguarding your Laravel application from common threats.
Input Validation and Sanitization
Effective input validation is essential for safeguarding Laravel applications from various attacks, including SQL injection and cross-site scripting (XSS). By rigorously validating user inputs, you ensure that the data processed by your application adheres to expected formats and values, thus preserving data integrity.
The Critical Role of Input Validation
Improperly validated input can be a gateway for malicious attacks:
- SQL Injection: Attackers can manipulate SQL queries, exploiting vulnerabilities to gain unauthorized access to the database.
- XSS Attacks: Malicious scripts can be injected into web pages viewed by other users, compromising sensitive information.
Leveraging Laravel’s Built-in Validation Rules
Laravel offers robust validation mechanisms that simplify the process of ensuring data integrity. Here are some key features:
- Form Requests: A dedicated class for handling form validation logic, providing a clean way to validate request data.
- Validator Facade: Allows defining custom validation rules with ease.
Example Usage:
php $request->validate([ ’email’ => ‘required|email’, ‘password’ => ‘required|min:8’, ]);
Laravel’s extensive list of built-in validation rules covers common requirements such as format constraints, value ranges, and more.
By utilizing these powerful tools, developers can significantly reduce the risk of injection attacks and enhance the overall security of their Laravel applications.
CSRF Protection in Laravel Applications
Cross-Site Request Forgery (CSRF) attacks exploit the trust that a web application has in a user’s browser. Malicious actors can trick users into executing unwanted actions on web applications where they are authenticated. This could lead to unauthorized transactions, data theft, or other harmful activities.
How do CSRF Attacks work?
- User Authentication: A user logs into a legitimate site (e.g., a banking site).
- Malicious Site: The user then visits a malicious site while still logged into the legitimate site.
- Forged Request: The malicious site sends a request to the legitimate site using the authenticated user’s session.
Such vulnerabilities can have severe implications, including financial loss and unauthorized access to sensitive information.
Utilizing the @csrf Directive
Laravel simplifies CSRF protection with its built-in middleware and the @csrf directive.
- Middleware Protection: Laravel includes CSRF protection middleware that automatically checks for tokens on every POST, PUT, PATCH, or DELETE request.
- Token Generation: In your Blade templates, use the @csrf directive to generate hidden input fields with tokens. This will help in demonstrating a Laravel app to submit as own code employers.
These tokens ensure that each form submission is unique and valid, safeguarding your application from potential CSRF attacks.
To stay secure against CSRF threats, regularly review your codebase and ensure all forms include the @csrf directive. This practice is crucial for maintaining robust security in your Laravel application. The potential employers want the Laravel app for where to find it for developers submitting an open-source Laravel project. Understanding CSRF protection is essential for secure coding practices in an open-source Laravel project to submit to potential employers.
Secure File Uploads Handling
Allowing users to upload files can pose significant security risks if not managed correctly. Malicious users might attempt to upload malware or exploit vulnerabilities to gain unauthorized access to sensitive data.
Key Risks:
- Malware distribution: Uploaded files could contain malicious code.
- Unauthorized access: Improper handling might lead to exposure of sensitive information. To mitigate these risks, it’s essential to implement robust file validation mechanisms.
Best Practices for Secure File Uploads
MIME Type Validation:
- Ensure the uploaded file’s MIME type matches the expected type.
- Use Laravel’s validation rules: php $request->validate([ ‘file’ => ‘required|mimes:jpeg,bmp,png|max:2048’, ]);
Size Restrictions:
- Limit the size of uploaded files to prevent resource exhaustion attacks.
- Example rule in Laravel: php $request->validate([ ‘file’ => ‘required|file|max:2048’, // Max size in kilobytes ]);
Storage Considerations:
- Store files outside the public directory to prevent direct access.
- Example configuration in filesystems.php: php ‘disks’ => [ ‘secure_uploads’ => [ ‘driver’ => ‘local’, ‘root’ => storage_path(‘app/secure_uploads’), ], ],
By following these best practices, you can significantly reduce the risk associated with file uploads, ensuring your application remains secure against potential threats.
Secure Management of Environment Variables in Laravel Projects
Managing sensitive information such as database credentials, API keys, and other configuration settings securely is vital for protecting your Laravel application. The .env file in a Laravel project is the primary place where such sensitive data is stored. These key files are not found an error in image manager storage craft.
Best Practices for .env file security
- Restrict Access: Ensure that the .env file has limited access permissions. Only essential users and processes should have read/write access to this file. shell chmod 600 .env
- Environment-Specific Configurations: Avoid hardcoding environment-specific configurations directly in your codebase. Instead, use environment variables to make your application more flexible and secure.
- Version Control Exclusion: Never commit your .env file to version control systems like Git. Add it to your .gitignore file to prevent accidental exposure. Plaintext
.gitignore
- Encryption of Sensitive Data: Encrypt sensitive environment variables if possible. Using packages like laravel-environment-manager can help manage encrypted environment variables securely.
- Dynamic Configuration Loading: Utilize services like AWS Secrets Manager or HashiCorp Vault to dynamically load sensitive configurations at runtime, reducing reliance on static files.
By integrating these practices, you enhance the protection of sensitive information within your Laravel applications, aligning with best practices in secure application development.
Session Management Techniques for Enhanced Security
Effective session management is crucial to maintaining the security and integrity of your Laravel applications. Properly configuring session settings can mitigate risks such as session fixation and hijacking attacks.
Configuring Secure Flags
Laravel provides various configuration options to enhance session security:
- Secure Cookie Flag: Ensure that the session.cookie_secure setting is enabled in config/session.php. This forces cookies to be sent over HTTPS connections only.
- HTTP Only Flag: By setting the http_only flag, you prevent JavaScript from accessing the cookie, reducing the risk of cross-site scripting (XSS) attacks.
- Same-Site Attribute: The same_site attribute helps protect against cross-site request forgery (CSRF) by ensuring cookies are not sent along with cross-site requests.
Session Lifetime Management
Adjusting session lifetimes can also contribute to security. Shorter session lifetimes minimize the window of opportunity for attackers to exploit stolen sessions.
php ‘lifetime’ => env(‘SESSION_LIFETIME’, 120), // in minutes
Session Fixation Prevention
Laravel automatically regenerates the session ID upon user authentication, helping prevent session fixation attacks. However, it’s a good practice to call Session::regenerate() manually during critical operations like password changes.
php use Illuminate\Support\Facades\Session;
Session::regenerate();
By implementing these practices, you can significantly enhance the security of your application’s session management and protect against common threats.
Rate Limiting Implementation in Laravel Applications
Rate limiting is crucial in preventing abuse or automated attacks, particularly on login routes and APIs. By controlling the number of requests a user can make within a certain timeframe, you can effectively mitigate brute-force attacks and other forms of abuse.
Implementing Rate Limiting in Laravel
Laravel provides built-in support for rate limiting via middleware. You can easily apply rate limiting to your routes by using the throttle middleware. Here’s how you can set it up:
1. Apply Throttle Middleware to Routes:
php Route::middleware(‘throttle:60,1’)->group(function () { Route::post(‘/login’, [LoginController::class, ‘login’]); Route::post(‘/register’, [RegisterController::class, ‘register’]); });
In this example, the throttle:60,1 middleware limits the number of requests to 60 per minute.
2. Customizing Rate Limits:
You may need different rate limits for different parts of your application. Customize them as needed:
php Route::middleware(‘throttle:10,1’)->group(function () { Route::post(‘/api/v1/resource’, [ApiController::class, ‘store’]); });
3. Dynamic Rate Limiting:
If you require dynamic rate limiting based on user roles or other conditions, you can define custom throttle classes:
php use Illuminate\Routing\Middleware\ThrottleRequests;
class CustomThrottle extends ThrottleRequests { protected function resolveRequestSignature($request) { return $request->user()->id ?: $request->ip(); } protected function resolveMaxAttempts($request) { return $request->user()->isPremium() ? 100 : 60; } }
Benefits of Rate Limiting
- Prevents Brute-Force Attacks: Limits repeated login attempts and API calls.
- Reduces Server Load: Protects server resources from being overwhelmed by excessive requests.
- Improves User Experience: Ensures fair usage among users by preventing abuse.
By implementing rate limiting, you enhance your application’s security posture and ensure a more stable and reliable environment for legitimate users.
Error Handling Best Practices for Production Environments
Effective error handling in production environments is crucial to maintain the security and stability of your Laravel application. Exposing sensitive debug information to end-users during error occurrences can pose significant risks, such as revealing underlying code structures, server configurations, or other critical information that attackers could exploit.
1. Disabling Debug Mode
One of the first steps you should take is disabling debug mode in your production environment. Laravel’s .env file contains a APP_DEBUG setting which should be set to false in production:
env APP_DEBUG=false
When APP_DEBUG is set to true, detailed error messages and stack traces are displayed, which can inadvertently expose vulnerabilities.
2. Custom Error Pages
Implementing custom error pages helps shield users from seeing raw error data. Laravel allows you to easily create custom views for different HTTP status codes by placing them in the resources/views/errors directory. For instance, a custom 404 error page can be created at:
plaintext resources/views/errors/404.blade.php
3. Logging Errors
Instead of displaying errors directly to users, log them for internal review and analysis. Laravel’s logging configuration can be customized through the config/logging.php file. Choose appropriate logging channels such as daily logs or external services like Sentry for monitoring and alerting.
4. Third-Party Error Tracking
Integrating third-party error tracking tools like Bugsnag or Sentry provides real-time monitoring and detailed reports on exceptions occurring in your application. These tools help you quickly identify and resolve issues without exposing sensitive information to the public.
By implementing these best practices, you significantly reduce the risks associated with improper error handling and ensure a more secure environment for your Laravel applications.
Conducting Regular Security Audits on Your Codebase
Regular security audits are essential for maintaining the integrity and security of your Laravel applications. By systematically evaluating your codebase, you can identify potential vulnerabilities and ensure compliance with best practices.
Tools and Techniques for Security Assessments
1. Static Code Analysis Tools:
- PHPStan: A powerful static analysis tool that helps detect bugs and enforce coding standards before deployment.
- Larastan: An extension of PHPStan specifically designed for Laravel projects, providing deeper insight into Laravel-specific issues.
2. Dependency Scanners:
- Composer Audit: This command checks your dependencies against known security vulnerabilities, ensuring that all packages are up-to-date and secure.
- Snyk: A comprehensive tool that scans for vulnerabilities in open source libraries and provides actionable remediation steps.
3. Penetration Testing:
Manual penetration testing involves simulating attacks to discover security weaknesses. Engaging third-party security experts can provide an unbiased assessment of your application’s defenses.
4. Automated Vulnerability Scanners:
- OWASP ZAP (Zed Attack Proxy): An easy-to-use tool that helps find security vulnerabilities in web applications during development and testing phases.
- Burp Suite: A robust platform for performing security testing of web applications, including a free community edition with essential features.
Best Practices for Security Audits
- Schedule regular audits, ideally after major code changes or before significant releases.
- Integrate automated tools into your CI/CD pipeline to catch issues early.
- Document findings and remediation actions to maintain a clear audit trail.
- Encourage peer code reviews with a focus on security aspects.
By leveraging these tools and techniques, you can systematically enhance the security posture of your Laravel applications and protect against potential threats.
SSL/TLS Encryption Setup for Secure Data Transmission Over HTTP(S) Connections
HTTPS configuration is crucial in protecting user data while it’s being transmitted. By enabling SSL/TLS encryption, you can keep sensitive information safe from being intercepted or modified.
Benefits of Enabling HTTPS
- Data Integrity: Ensures that data sent between the client and server remains unchanged.
- Privacy and Security: Encrypts the data, making it unreadable to anyone who tries to intercept the traffic.
- Trust and SEO: Increases user trust and improves search engine rankings since search engines prefer sites that use HTTPS.
Steps to Enable HTTPS in Laravel
- Obtain an SSL Certificate: Purchase or get a free SSL certificate from providers like Let’s Encrypt.
- Install the Certificate on Your Web Server: For Apache: bash sudo a2enmod ssl sudo a2ensite default-ssl.conf sudo service apache2 reload
For Nginx: bash server { listen 443 ssl; server_name yourdomain.com;
ssl_certificate /path/to/certificate.crt;
ssl_certificate_key /path/to/private.key;
# Other configurations…
} - Update Laravel Configuration: Make sure Laravel uses HTTPS by updating config/app.php: php ‘url’ => env(‘APP_URL’, ‘https://yourdomain.com’),
- Force HTTPS with Middleware: Use Laravel’s built-in middleware to redirect all HTTP requests to HTTPS: php protected $middleware = [ // Other middleware… \App\Http\Middleware\RedirectIfAuthenticated::class, ];
By setting up SSL/TLS encryption, you significantly enhance the security of your Laravel applications, ensuring that data is transmitted securely over HTTP(S) connections.
Access Control Policies Implementation Using Roles & Permissions Management System
Access control policies in Laravel are crucial for ensuring that users have appropriate permissions to access various parts of your application. Leveraging Laravel’s built-in authorization features allows you to enforce granular access control effectively.
Role-Based Access Control (RBAC)
Laravel source code tools are best for implementing role-based access control (RBAC). With these open source code Laravel used tools, you can easily manage roles and permissions.
1. Defining Roles and Permissions:
php use Spatie\Permission\Models\Role; use Spatie\Permission\Models\Permission;
$role = Role::create([‘name’ => ‘admin’]); $permission = Permission::create([‘name’ => ‘edit articles’]);
$role->givePermissionTo($permission);
2. Assigning Roles to Users:
php use App\Models\User;
$user = User::find(1); $user->assignRole(‘admin’);
Middleware for Authorization
Laravel also supports middleware for authorization, allowing you to restrict access to specific routes based on roles or permissions:
php Route::group([‘middleware’ => [‘role:admin’]], function () { Route::get(‘/dashboard’, [DashboardController::class, ‘index’]); });
Policy Classes
For more complex authorization logic, Laravel’s policy classes offer a way to organize and manage your authorization logic systematically:
1. Generating a Policy:
bash php artisan make:policy ArticlePolicy –model=Article
2. Defining Methods in the Policy:
php public function update(User $user, Article $article) { return $user->id === $article->user_id; }
3. Registering the Policy:
php protected $policies = [ ‘App\Models\Article’ => ‘App\Policies\ArticlePolicy’, ];
By implementing these techniques, you create a secure environment that ensures only authorized users can perform actions within your Laravel application. In Laravel tutorials, creating a useful tool builds up robust web applications.
Conclusion
Staying vigilant and updated with the latest security practices is crucial for securing Laravel applications. Regularly monitoring your application’s vulnerability landscape ensures that potential threats are identified and mitigated promptly. By adopting these proactive measures, you’re well-equipped to maintain a robust defense against various security threats in your Laravel applications.