5 Simple Steps to Get Raw SQL Queries with Laravel Builder
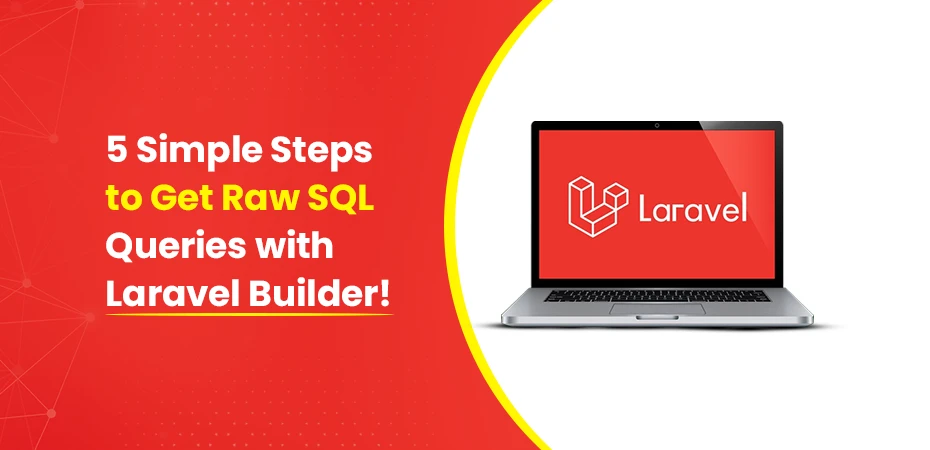
Have you ever wondered what SQL queries your Laravel application is actually running behind the scenes? Understanding these queries is crucial for debugging, performance optimization, and ensuring your database interactions work as intended.
Laravel’s Query Builder and Eloquent ORM are powerful tools that simplify database operations. They abstract away complex SQL syntax, making database interactions more intuitive and maintainable. Yet, there are times when you need to peek under the hood and see how with the help of laravel query builder, SQL’s raw queries are being executed.
Think of Laravel’s Query Builder for raw SQL queries as your personal SQL translator:
- It converts your PHP method chains into SQL statements
- It handles database security through query bindings
- It manages complex relationships with minimal code
Why check Laravel’s raw SQL queries?
- Debug unexpected query results
- Optimize database table performance
- Understand query execution patterns
- Validate security measures like proper parameter binding
This guide will show you five easy ways to get and analyze raw SQL queries in your Laravel applications. Whether you’re optimizing performance, handling complex joins, or integrating legacy databases, knowing how laravel runs a raw SQL query in Entity Framework can be invaluable.
In Entity Framework, raw SQL queries parameters allow you to bypass the Entity Framework’s LINQ queries and work directly with SQL statements.
Step 1: Using the toSql() Method
The toSql() method is your go-to tool for peeking under the hood of the Query Builder in Laravel. This handy method reveals the raw SQL query string before it’s executed, giving you a clear view of what’s happening in your database interactions.
Let’s look at a practical example:
php $sql = User::where(‘created_at’, ‘<‘, now()->subYear())->toSql(); dd($sql);
This code snippet generates a SQL query like this:
sql select * from users where created_at < ?
Key Features of toSql():
- Returns the query as a plain string
- Shows query structure with parameter placeholders
- Works with both Eloquent and Query Builder
- Doesn’t execute the actual query
You can chain toSql() with various query methods:
php
// With multiple conditions
$sql = User::where(‘active’, 1) ->where(‘role’, ‘admin’) ->orderBy(‘created_at’) ->toSql();
// With joins
$sql = User::join(‘posts’, ‘users.id’, ‘=’, ‘posts.user_id’) ->select(‘users.*’, ‘posts.title’) ->toSql();
Important Note: The toSql() method displays question marks (?) as placeholders instead of actual values. This reflects Laravel’s use of prepared statements for security. These placeholders represent the values that would be bound to the query during execution.
The raw query output helps you:
- Debug complex queries
- Verify query structure
- Optimize database system operations
- Understand Laravel’s query building process
Step 2: Retrieving Bindings with getBindings()
SQL query bindings are essential for keeping your Laravel application safe from SQL injection attacks and improving query performance. Let’s explore how to retrieve these bindings effectively.
Understanding getBindings()
The getBindings() method reveals the actual values that replace the question mark placeholders in your SQL queries. Here’s a practical example:
php $query = User::where(’email’, ‘john@example.com’) ->where(‘status’, ‘active’);
$bindings = $query->getBindings(); // Output: [‘john@example.com’, ‘active’]
Combining toSql() and getBindings()
To get a complete picture of your query execution, combine toSql() with getBindings():
php $query = User::where(‘created_at’, ‘<‘, now()->subYear()); $sql = $query->toSql(); $bindings = $query->getBindings();
// Create a function to replace placeholders with actual values function getSqlWithBindings($sql, $bindings) { return vsprintf(str_replace(‘?’, ‘%s’, $sql), $bindings); }
$fullQuery = getSqlWithBindings($sql, $bindings);
This approach helps you:
- Debug complex queries by seeing the exact values used
- Validate parameter binding before query execution
- Track data flow through your application’s database layer
Logging Queries with Actual Values
You can also use this method to log queries with their actual values:
php Log::info(‘Query:’, [ ‘sql’ => $sql, ‘bindings’ => $bindings, ‘full_query’ => $fullQuery ]);
Remember that binding values automatically escape special characters and handle data type conversion, making your queries both secure and reliable.
Step 3: Enabling Query Log for Detailed Insights
Laravel’s query logging feature provides a powerful way to track and analyze database queries during development. Let’s dive into how you can leverage this functionality for detailed debugging insights.
How to Enable and Retrieve Query Logs
Here’s how to enable and retrieve query logs in your Laravel application:
php // Enable query logging DB::enableQueryLog();
// Execute your database queries User::where(‘created_at’, ‘<‘, now()->subYear())->get();
// Get the log of all executed queries $queries = DB::getQueryLog();
Understanding the Query Log Output
The getQueryLog() method returns an array containing detailed information about each executed query:
- SQL Statement: The raw SQL query string
- Query Bindings: Parameter values used in the query
- Execution Time: Time taken to execute the query in milliseconds
Use Cases for Query Logging
This logging mechanism proves invaluable in several debugging scenarios:
- Performance Optimization: Identify slow queries and potential bottlenecks
- N+1 Query Detection: Spot redundant database calls in your application
- Data Integrity Verification: Ensure queries are executing with correct parameters
- Development Debugging: Track unexpected query behavior during development
Analyzing Query Log Output
You can iterate over the query log to analyze its output. Here’s an example of how to do that:
php // Example of analyzing query log output foreach (DB::getQueryLog() as $query) { dump([ ‘sql’ => $query[‘query’], ‘bindings’ => $query[‘bindings’], ‘time’ => $query[‘time’] ]); }
Disabling Query Logging in Production
Remember to disable query logging in production environments as it consumes additional memory:
php DB::disableQueryLog();
Enabling Query Logging in Development
For development environments, you can enable query logging in your AppServiceProvider to automatically track queries across your entire application:
php if (App::environment(‘local’)) { DB::enableQueryLog(); }
Step 4: Listening for Database Events
Database event listeners give you real-time insights into query execution. Let’s explore how to set up these listeners for powerful query monitoring.
Setting Up Event Listeners
You can register database event listeners in your AppServiceProvider.php file. Add this code to the boot() method:
php DB::listen(function ($query) { Log::info($query->sql); Log::info($query->bindings); Log::info($query->time); });
This listener captures three key pieces of information:
- The raw SQL query
- Parameter bindings
- Query execution time in milliseconds
Advanced Monitoring Options
You can customize the listener to track specific query patterns:
php DB::listen(function ($query) { if ($query->time > 100) { Log::warning(‘Slow query detected:’, [ ‘sql’ => $query->sql, ‘bindings’ => $query->bindings, ‘time’ => $query->time ]); } });
Real-World Applications
Database event listeners serve multiple purposes:
- Performance Monitoring: Track slow queries affecting application speed
- Security Auditing: Log sensitive data access patterns
- Debug Complex Issues: Identify problematic queries during development
- Query Optimization: Analyze query patterns for potential improvements
You can extend the listener functionality by sending notifications:
php DB::listen(function ($query) { if (str_contains($query->sql, ‘DELETE’)) { Notification::route(‘slack’, ‘webhook-url’) ->notify(new QueryAlert($query)); } });
This monitoring approach works seamlessly with both Query Builder and Eloquent operations, providing comprehensive visibility into your application’s database interactions.
Step 5: Debugging Laravel Application Query API with Tools like Debugbar and Telescope
Laravel’s ecosystem offers powerful debugging tools that make SQL query inspection a breeze. Let’s explore these essential development companions:
Laravel Debugbar
Debugbar is a lightweight implementation that provides an in-page debugging interface, focusing on request-specific data. It is ideal for local development
Key Features:
- Real-time query monitoring
- Query execution time tracking
- Memory usage statistics
- Request and response data display
To install Debugbar, run the following command:
php composer require barryvdh/laravel-debugbar –dev
The Debugbar appears as a sleek panel at the bottom of your browser, displaying executed queries with syntax highlighting and formatting. You can expand each query to view:
- Raw SQL statements
- Query bindings
- Execution time
- Stack trace
Laravel Telescope
Telescope is a more advanced debugging tool that offers persistent data storage and historical request tracking. It also provides advanced filtering options, making it better suited for staging environments.
Advanced Debugging Capabilities:
- Comprehensive request monitoring
- Database query inspection
- Cache operation tracking
- Job and event monitoring
To install Telescope, run the following commands:
php composer require laravel/telescope –dev php artisan telescope:install
Telescope provides a dedicated dashboard at /telescope with detailed insights into your application’s database interactions.
Debugbar vs Telescope Comparison
Here’s a comparison between Debugbar and Telescope to help you decide which one to use:
Feature | Debugbar | Telescope |
---|---|---|
Lightweight | Yes | No |
Persistent | No | Yes |
Debugging Interface | In-page | Web dashboard |
Data Focus | Request-specific | Historical |
Ideal Usage | Local development | Staging environments |
Tinkerwell Integration
Tinkerwell offers a graphical interface for query testing:
php User::where(‘active’, true)->dd(); // Displays query in Tinkerwell
These tools eliminate the need for manual query logging and provide rich debugging experiences with minimal setup requirements.
Conclusion
Debugging and optimizing the Laravel database select type is vital for ensuring application efficiency. These five methods give you powerful tools to peek under the hood of your database operations. Each technique serves a unique purpose – from quick SQL checks with toSql() to comprehensive monitoring through event listeners.
Ready to take your Laravel development to the next level? Our dedicated Laravel developers bring years of experience in performance optimization, query debugging, and database architecture. Let our experts handle the complex database operations while you focus on growing your business.
We’ve helped countless teams streamline their development process and build robust Laravel applications. Let our experts handle the complex database operations while you focus on growing your business.
Frequently Asked Questions (FAQs)
Using methods like toSql() or getBindings() has minimal impact on performance. These methods are typically used during development and debugging, not in production code.
It’s recommended to avoid enabling query logging in production. Use Laravel Telescope with restricted access or implement conditional logging based on your environment settings.
Yes, all the methods discussed work with Eloquent relationships. You can chain toSql() or enable query logging to see the generated queries for relationship operations.
toSql() shows the query structure without executing it, while DB::listen captures actual executed queries with their bindings and execution times.
Yes, these methods work across all database drivers supported by Laravel, including MySQL, PostgreSQL, SQLite, and SQL Server.